python opencv多线程处理图片
时间: 2024-07-12 13:01:28 浏览: 169
在Python中,结合OpenCV(Open Source Computer Vision Library)和多线程可以有效地并行处理大量图像数据,提高程序的执行效率。这是因为OpenCV本身并不是设计为多线程友好的,但它可以与Python的多线程或多进程库如`threading`或`multiprocessing`配合使用。
以下是使用多线程处理OpenCV图像的一般步骤:
1. **导入必要的模块**:
```python
import cv2
import threading
```
2. **定义读取、预处理或分析图像的方法**:
```python
def process_image(image):
# 在这里编写对图像的操作,例如缩放、裁剪或特征检测等
pass
```
3. **创建线程池**:
```python
image_threads = []
images_to_process = [...] # 需要处理的图像列表
for image in images_to_process:
thread = threading.Thread(target=process_image, args=(image,))
image_threads.append(thread)
thread.start() # 启动线程
```
4. **等待所有线程完成**:
```python
for thread in image_threads:
thread.join()
```
5. **注意同步问题**:
- 当多个线程同时访问共享资源时,可能会导致竞态条件,需谨慎管理线程间的数据访问。
- 使用互斥锁 (`threading.Lock()`) 或其他同步机制来保证数据一致性。
6. **可选:结果收集与合并**(如果需要的话)。
阅读全文
相关推荐
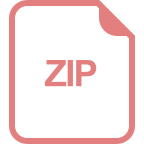
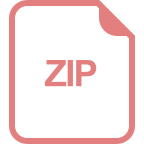
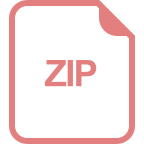





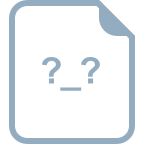
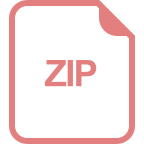
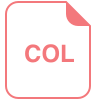
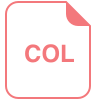
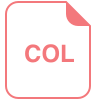
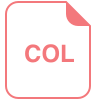




