python中使用opencv对多个图像进行多线程处理
时间: 2024-01-18 22:00:30 浏览: 62
在Python中使用OpenCV对多个图像进行多线程处理可以使用Python的threading模块来实现。具体步骤如下:
1. 导入必要的模块:首先需要导入OpenCV和threading模块。
```python
import cv2
import threading
```
2. 创建线程类:使用threading模块的Thread类来创建一个用于处理图像的线程类,并在类的构造函数中传入需要处理的图像。
```python
class ImageProcessingThread(threading.Thread):
def __init__(self, image):
threading.Thread.__init__(self)
self.image = image
def run(self):
# 在这里进行图像处理的逻辑
# 可以使用OpenCV的API来处理图像
# self.image表示当前线程处理的图像
```
3. 创建线程并启动:创建多个ImageProcessingThread线程对象,并使用start()方法来启动线程。
```python
# 假设有三个图像需要处理,分别为image1、image2、image3
# 创建线程对象
thread1 = ImageProcessingThread(image1)
thread2 = ImageProcessingThread(image2)
thread3 = ImageProcessingThread(image3)
# 启动线程
thread1.start()
thread2.start()
thread3.start()
# 等待所有线程执行完毕
thread1.join()
thread2.join()
thread3.join()
```
通过以上步骤,可以实现对多个图像同时进行多线程处理。每个线程在run()方法中可以使用OpenCV的API来读取、处理和保存图像。注意在使用多线程的时候,要注意线程之间的同步和资源的互斥访问,以确保程序的正确性。
相关推荐
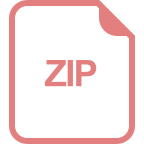
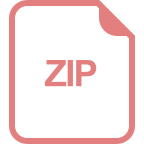
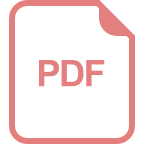
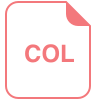
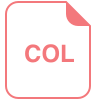
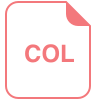
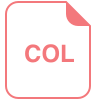
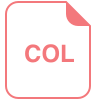









