根据自身对面向对象方法与系统设计的理解,参照《重构-改善既有代码的设计》书中关于不良设计(违反SOLID)、不良代码(代码坏味道bad smells)的判别标准进行重构int Library::indexOfNum(string num) { int i; for (i = 0; i < books.size(); i++) { if (books[i]->getNum() == num) return i; } return -1; } int Library::looknum(string num) { int i; for (i = 0; i < readers.size(); i++) { if (readers[i].getadress() == num) return i; } return -1; }void Library::filemanage() { fstream f; string s; f.open("books.txt", ios::out); addBook(); for (int i = 0; i < books.size(); i++) { f << books[i]->getName() << " " << books[i]->getAuther() << " " << books[i]->getNum() << " " << books[i]->getinstock() << endl; } f.close(); cout << "输入成功" << endl; } void Library::open() { fstream f; f.open("books.txt", ios::in); string name; string auther; string number; int instock; while (f >> name >> auther >> number >> instock) { cout << name << " " << auther << " " << number << " " << instock << endl; int i; for (i = 0; i < books.size(); i++) { if (books[i]->getNum() == number) { books[i]->setname(name); books[i]->setauther(auther); books[i]->setnum(number); books[i]->set(instock); } } } f.close(); cout << "输出成功" << endl; system("pause"); system("cls"); }
时间: 2024-03-18 18:40:29 浏览: 122
这段代码存在以下不良设计和不良代码:
1. 单一职责原则(SRP)违反:`Library` 类的 `indexOfNum()` 和 `looknum()` 函数分别负责查找图书和读者,职责不够单一。
2. 开闭原则(OCP)违反:如果需要增加新的查找方法,需要修改 `Library` 类,导致代码不够灵活。
3. 依赖倒置原则(DIP)违反:`Library` 类直接依赖于具体的 `fstream` 类和 `Book` 类,限制了代码的可扩展性和可维护性。
4. 代码坏味道:函数名不够明确,不符合命名规范。变量名 `i`、`s`、`f`、`num` 等也不够明确。
为了重构这段代码,可以采取以下步骤:
1. 根据 SRP 原则,将 `indexOfNum()` 和 `looknum()` 函数分别拆分成两个函数,分别用于查找图书和读者。
2. 根据 OCP 原则,将查找方法进行抽象,定义一个 `Searchable` 接口,不同类型的查找实现该接口,然后在 `Library` 类中使用该接口。
3. 根据 DIP 原则,将具体的 `fstream` 类和 `Book` 类依赖抽象出来,使用依赖注入的方式传入 `Library` 类中。
4. 重命名函数和变量,使其更加清晰明了。
重构后的代码如下:
```cpp
class Searchable {
public:
virtual ~Searchable() {}
virtual int search(const string& query) = 0;
};
class BookNumSearch : public Searchable {
public:
BookNumSearch(vector<unique_ptr<Book>>& books) : books(books) {}
int search(const string& num) override {
for (int i = 0; i < books.size(); i++) {
if (books[i]->getNum() == num) {
return i;
}
}
return -1;
}
private:
vector<unique_ptr<Book>>& books;
};
class ReaderAddressSearch : public Searchable {
public:
ReaderAddressSearch(vector<Reader>& readers) : readers(readers) {}
int search(const string& address) override {
for (int i = 0; i < readers.size(); i++) {
if (readers[i].getAddress() == address) {
return i;
}
}
return -1;
}
private:
vector<Reader>& readers;
};
class Library {
public:
Library(fstream& file, vector<unique_ptr<Book>>& books, vector<Reader>& readers)
: file(file), books(books), readers(readers) {}
void writeToFile() {
file.open("books.txt", ios::out);
for (const auto& book : books) {
file << book->getName() << " " << book->getAuthor() << " "
<< book->getNum() << " " << book->getInStock() << endl;
}
file.close();
cout << "输入成功" << endl;
}
void open() {
file.open("books.txt", ios::in);
string name;
string author;
string num;
int inStock;
while (file >> name >> author >> num >> inStock) {
cout << name << " " << author << " " << num << " " << inStock << endl;
int index = bookNumSearch.search(num);
if (index != -1) {
books[index]->setName(name);
books[index]->setAuthor(author);
books[index]->setNum(num);
books[index]->setInStock(inStock);
}
}
file.close();
cout << "输出成功" << endl;
system("pause");
system("cls");
}
void addBook(unique_ptr<Book> book) {
books.push_back(move(book));
}
void addReader(const Reader& reader) {
readers.push_back(reader);
}
private:
fstream& file;
vector<unique_ptr<Book>>& books;
vector<Reader>& readers;
BookNumSearch bookNumSearch { books };
ReaderAddressSearch readerAddressSearch { readers };
};
```
重构后的代码更符合 SOLID 原则,可扩展性和可维护性更好,并且避免了不良代码坏味道。
阅读全文
相关推荐



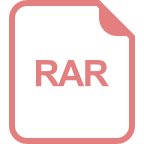


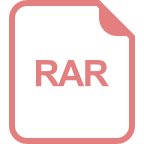







