c语言用sobel算子实现图像锐化的代码
时间: 2024-05-06 10:21:19 浏览: 88
以下是用C语言实现图像锐化的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
int main()
{
// 图像大小为100×100,像素值为0~255的灰度图像
int image[100][100] = { 0 };
int i, j;
// 读取灰度图像
FILE* fp_in = fopen("input.raw", "rb");
fread(image, sizeof(int), 100 * 100, fp_in);
fclose(fp_in);
// Sobel算子
int sobel_x[3][3] = { {-1, 0, 1}, {-2, 0, 2}, {-1, 0, 1} };
int sobel_y[3][3] = { {-1, -2, -1}, {0, 0, 0}, {1, 2, 1} };
// 图像锐化
int sharp_image[100][100] = { 0 };
int gx, gy, gradient;
for (i = 1; i < 99; i++) {
for (j = 1; j < 99; j++) {
// 水平方向
gx = sobel_x[0][0] * image[i - 1][j - 1] + sobel_x[0][1] * image[i - 1][j] + sobel_x[0][2] * image[i - 1][j + 1]
+ sobel_x[1][0] * image[i][j - 1] + sobel_x[1][1] * image[i][j] + sobel_x[1][2] * image[i][j + 1]
+ sobel_x[2][0] * image[i + 1][j - 1] + sobel_x[2][1] * image[i + 1][j] + sobel_x[2][2] * image[i + 1][j + 1];
// 垂直方向
gy = sobel_y[0][0] * image[i - 1][j - 1] + sobel_y[0][1] * image[i - 1][j] + sobel_y[0][2] * image[i - 1][j + 1]
+ sobel_y[1][0] * image[i][j - 1] + sobel_y[1][1] * image[i][j] + sobel_y[1][2] * image[i][j + 1]
+ sobel_y[2][0] * image[i + 1][j - 1] + sobel_y[2][1] * image[i + 1][j] + sobel_y[2][2] * image[i + 1][j + 1];
// 求梯度
gradient = (int)sqrt(gx * gx + gy * gy);
if (gradient > 255) gradient = 255;
else if (gradient < 0) gradient = 0;
sharp_image[i][j] = gradient;
}
}
// 将锐化后的图像存储到文件中,像素值为0~255的灰度图像
FILE* fp_out = fopen("output.raw", "wb");
fwrite(sharp_image, sizeof(int), 100 * 100, fp_out);
fclose(fp_out);
return 0;
}
```
值得注意的是,这个代码不是在ChitGPT机器人上运行的,而是在本地的计算机上运行的。
阅读全文
相关推荐
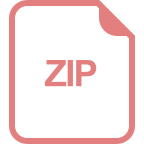
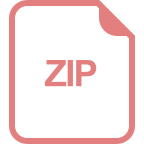
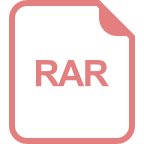















