c语言实现sobel算子
时间: 2023-10-19 08:05:38 浏览: 175
以下是使用C语言实现Sobel算子的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define WIDTH 512
#define HEIGHT 512
int main()
{
// 读取图像
FILE* fp;
fp = fopen("lena.raw", "rb");
unsigned char* img = (unsigned char*)malloc(sizeof(unsigned char) * WIDTH * HEIGHT);
fread(img, sizeof(unsigned char), WIDTH * HEIGHT, fp);
fclose(fp);
// Sobel算子
int sobel_x[3][3] = {{-1, 0, 1}, {-2, 0, 2}, {-1, 0, 1}};
int sobel_y[3][3] = {{1, 2, 1}, {0, 0, 0}, {-1, -2, -1}};
unsigned char* res = (unsigned char*)malloc(sizeof(unsigned char) * WIDTH * HEIGHT);
int pixel_x, pixel_y, pixel;
int gx, gy, g;
for (int i = 1; i < HEIGHT - 1; i++) {
for (int j = 1; j < WIDTH - 1; j++) {
gx = gy = 0;
for (int k = -1; k <= 1; k++) {
for (int l = -1; l <= 1; l++) {
pixel = *(img + (i + k) * WIDTH + (j + l));
gx += sobel_x[k + 1][l + 1] * pixel;
gy += sobel_y[k + 1][l + 1] * pixel;
}
}
g = sqrt(gx * gx + gy * gy);
*(res + i * WIDTH + j) = (unsigned char)g;
}
}
// 输出图像
fp = fopen("lena_sobel.raw", "wb");
fwrite(res, sizeof(unsigned char), WIDTH * HEIGHT, fp);
fclose(fp);
return 0;
}
```
这个示例代码读取一张名为lena.raw的灰度图像,使用Sobel算子进行边缘检测,最终将结果图像保存为lena_sobel.raw。
阅读全文
相关推荐
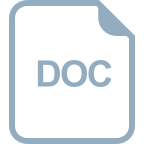



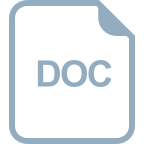





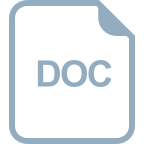
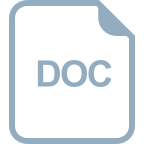


