Sobel算子用c语言
时间: 2024-06-09 16:06:06 浏览: 127
Sobel算子是一种常用的边缘检测算法,可以用于图像处理中。下面是使用C语言实现Sobel算子的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
int main()
{
// 定义Sobel算子
int SobelX[3][3] = {{-1, 0, 1}, {-2, 0, 2}, {-1, 0, 1}};
int SobelY[3][3] = {{-1, -2, -1}, {0, 0, 0}, {1, 2, 1}};
// 读取图像
FILE *fp = fopen("lena.raw", "rb");
if (fp == NULL) {
printf("Error: cannot open file!\n");
return -1;
}
unsigned char *img = (unsigned char*)malloc(sizeof(unsigned char) * 512 * 512);
fread(img, sizeof(unsigned char), 512 * 512, fp);
fclose(fp);
// 进行Sobel算子处理
int width = 512, height = 512;
unsigned char *result = (unsigned char*)malloc(sizeof(unsigned char) * width * height);
for (int i = 1; i < height - 1; i++) {
for (int j = 1; j < width - 1; j++) {
// 计算x方向和y方向的梯度
int gx = 0, gy = 0;
for (int k = -1; k <= 1; k++) {
for (int l = -1; l <= 1; l++) {
gx += SobelX[k+1][l+1] * img[(i+k)*width+(j+l)];
gy += SobelY[k+1][l+1] * img[(i+k)*width+(j+l)];
}
}
// 计算梯度幅值
int gradient = sqrt(gx*gx + gy*gy);
if (gradient > 255) gradient = 255;
result[i*width+j] = (unsigned char)gradient;
}
}
// 保存结果图像
fp = fopen("lena_sobel.raw", "wb");
if (fp == NULL) {
printf("Error: cannot open file!\n");
return -1;
}
fwrite(result, sizeof(unsigned char), width * height, fp);
fclose(fp);
free(img);
free(result);
return 0;
}
```
这段代码实现了对“lena.raw”图像的Sobel算子处理,并将结果保存为“lena_sobel.raw”文件。你可以根据自己的需要修改代码中的图像文件名、大小等参数。
阅读全文
相关推荐


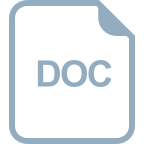
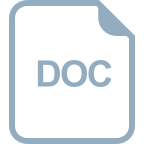






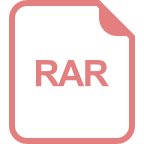
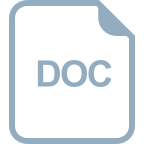
