写一段取指的verilog代码
时间: 2023-12-01 14:14:36 浏览: 99
以下是一段取指的Verilog代码:
module fetch(
input wire clk, // 时钟信号
input wire reset, // 复位信号
input wire [31:0] pc, // 程序计数器
output reg [31:0] inst // 输出指令
);
// 初始化PC值
reg [31:0] pc_reg = 32'h0;
always @(posedge clk) begin
if (reset) begin
// 复位信号为1时,将PC值重置为0
pc_reg <= 32'h0;
end
else begin
// 从pc_reg中读取指令
inst <= $readmemh("instruction_memory.txt", pc_reg >> 2);
// PC加4
pc_reg <= pc_reg + 32'h4;
end
end
endmodule
这段代码实现了一个简单的取指模块。在时钟上升沿时,如果复位信号为1,则将PC值重置为0;否则,从指令存储器中读取PC指向的指令,并将PC加4。指令存储器的内容需要在外部指定,这里假设指令存储器的内容已经保存在instruction_memory.txt文件中。
相关问题
用Verilog HDL语言实现取指电路
好的,我会尝试回答你的问题。
Verilog HDL是一种硬件描述语言,可以用来描述数字电路的行为和结构。取指电路是计算机中非常重要的一部分,它负责从存储器中读取指令,然后将指令送到译码器中进行解码。下面是一个简单的Verilog HDL代码,用于实现取指电路:
```
module instruction_fetch(
input clk,
input reset,
input [15:0] program_counter,
output [15:0] instruction
);
reg [15:0] memory [0:65535];
reg [15:0] next_program_counter;
initial begin
// 初始化存储器
$readmemh("program.hex", memory);
end
always @(posedge clk) begin
if (reset) begin
// 复位
next_program_counter <= 16'h0000;
end else begin
// 读取指令
instruction <= memory[program_counter];
next_program_counter <= program_counter + 16'h0001;
end
end
assign program_counter = next_program_counter;
endmodule
```
以上代码中,我们定义了一个名为“instruction_fetch”的模块,其中包含4个端口:时钟clk、复位信号reset、程序计数器program_counter和指令instruction。在模块内部,我们定义了一个存储器数组memory,用于存储程序的指令。在初始化阶段,我们使用$readmemh函数从一个.hex文件中读取程序,并将其存储到存储器中。
在时钟上升沿的时候,我们检查复位信号是否为1。如果为1,我们将下一个程序计数器next_program_counter设置为0,以便重新从程序的第一条指令开始执行。如果复位信号为0,我们通过program_counter读取存储器中的指令,并将下一个程序计数器设置为program_counter + 1。
最后,我们使用assign语句将program_counter连接到next_program_counter,以便在下一个时钟周期中更新程序计数器的值。
当然,这只是一个简单的Verilog HDL代码示例,实际的取指电路可能更加复杂。但是,这个示例可以帮助你了解如何使用Verilog HDL来实现取指电路。
五级流水线设计verilog代码
### 回答1:
五级流水线是一种常见的计算机系统设计技术,流水线可以将一个指令的执行过程分解为多个阶段,并且不同的指令可以同时在不同的阶段上执行,从而提高指令的执行效率。以下是一个基于Verilog代码的五级流水线设计的简单示例:
```verilog
module FiveStagePipeline(
input wire clk,
input wire [31:0] instruction,
output wire [31:0] result
);
reg [4:0] stage;
reg [31:0] pc, alu_a, alu_b, alu_result;
reg [2:0] opcode;
always @(posedge clk) begin
case(stage)
0: begin //第一阶段:取指令
pc <= pc + 4;
opcode <= instruction[31:29];
stage <= 1;
end
1: begin //第二阶段:译码
case(opcode)
3'b000: begin //算术指令
alu_a <= instruction[27:23];
alu_b <= instruction[22:18];
stage <= 2;
end
// 其他指令的译码逻辑
default: stage <= 0; //未知指令,回到第一阶段
endcase
end
2: begin //第三阶段:执行
case(opcode)
3'b000: begin //算术指令
alu_result <= alu_a + alu_b;
stage <= 3;
end
// 其他指令的执行逻辑
endcase
end
3: begin //第四阶段:访存
case(opcode)
3'b000: begin //算术指令
// 内存访问逻辑
stage <= 4;
end
// 其他指令的访存逻辑
endcase
end
4: begin //第五阶段:写回
case(opcode)
3'b000: begin //算术指令
result <= alu_result;
end
// 其他指令的写回逻辑
endcase
stage <= 0; //回到第一阶段
end
endcase
end
endmodule
```
在这个代码中,五级流水线被划分为取指令、译码、执行、访存和写回这五个阶段。每个时钟周期,根据当前所处的阶段,进行相应的指令处理操作。不同的指令执行逻辑可以根据具体需求进行编写。每个阶段都将指令的数据传递给下一个阶段,以实现流水线的连续执行。
### 回答2:
第一个步骤是设计五级流水线的结构。在设计中,我们需要确定流水线的五个阶段,并确保它们按顺序运行。
第一阶段是取指令(Instruction Fetch),它从存储器中获取指令并将其送入下一个阶段。
第二阶段是指令译码(Instruction Decode),它对指令进行解码并提取出操作码和操作数。它还可以根据需要进行寄存器读取和其他操作。
第三阶段是执行(Execute),它执行指令指定的操作,并根据需要计算结果。
第四阶段是访存(Memory Access),如果指令需要访问内存,则在该阶段进行读取或写入操作。
第五阶段是写回(Write Back),它将结果写回寄存器文件。
接下来,我们需要用Verilog代码实现这个五级流水线的结构。以下是一个简单的示例代码:
```verilog
module five_stage_pipeline (
input wire clk,
input wire reset,
input wire [31:0] instruction_in,
output wire [31:0] result_out
);
reg [31:0] instruction, result;
reg [3:0] state;
always @(posedge clk or posedge reset) begin
if (reset)
state <= 0;
else begin
case (state)
0: begin // Instruction Fetch
instruction <= instruction_in;
state <= 1;
end
1: begin // Instruction Decode
// Decode and extract opcode and operands
// Perform register read
state <= 2;
end
2: begin // Execute
// Perform necessary calculations
state <= 3;
end
3: begin // Memory Access
// Perform memory read or write if needed
state <= 4;
end
4: begin // Write Back
// Write result back to register file
state <= 0;
end
endcase
end
end
always @(posedge clk) begin
case (state)
2: begin // Execute
// Perform necessary calculations and assign result
end
4: begin // Write Back
// Assign result to output
result_out <= result;
end
endcase
end
endmodule
```
上述代码使用了一个有限状态机来管理流水线的阶段顺序,以确保每个阶段的操作按顺序进行。需要根据具体的需求和指令集进行适当的修改和扩展。
### 回答3:
五级流水线是一种常用的计算机设计技术,用于提高计算机的执行效率。在设计五级流水线时,我们需要使用Verilog编程语言来描述电路的行为和结构。
首先,我们需要定义五个阶段的流水线,分别是取指阶段(IF)、译码阶段(ID)、执行阶段(EX)、访存阶段(MEM)和写回阶段(WB)。
接下来,我们需要定义流水线寄存器,用于传递每个阶段的输出结果。我们可以使用Verilog中的寄存器类型来实现这些流水线寄存器。
然后,我们需要定义指令集和对应的操作码,根据操作码来执行对应的操作。在译码阶段,我们根据指令的操作码解析指令,并确定要执行的操作。
在执行阶段,我们需要实现各种指令的执行逻辑,例如加法、减法、乘法等。我们可以使用ALU(算术逻辑单元)来实现这些操作。
在访存阶段,我们会访问内存,读取或写入数据。我们可以使用Verilog中的存储单元类型(例如ram)来实现内存的读写操作。
最后,在写回阶段,我们将执行结果写回到寄存器中,以便后续使用。
整个五级流水线的设计需要考虑各个阶段的数据依赖关系和控制冒险的解决方法,例如前一条指令的结果是否会对后一条指令的执行有影响,以及如何解决数据冲突等问题。
总结来说,设计五级流水线的Verilog代码需要定义五个阶段的流水线以及对应的寄存器,实现指令的译码、执行、访存和写回,解决数据依赖和控制冒险等问题。这样可以提高计算机的执行效率和性能。
阅读全文
相关推荐
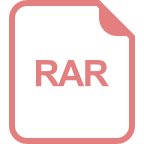
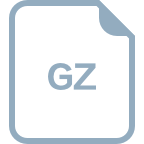
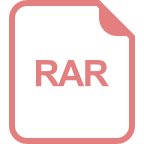
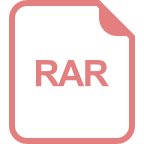
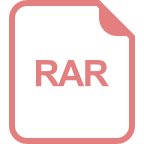
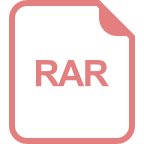
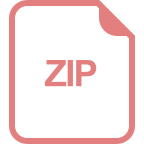
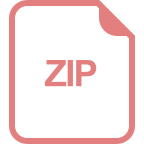
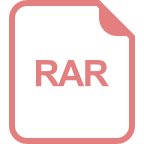
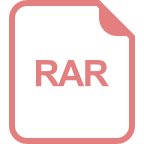
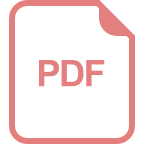
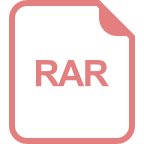
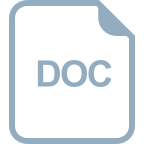