写出安卓怎么读取uvc摄像头上的按键事件
时间: 2023-12-05 19:04:25 浏览: 186
在安卓中读取UVC摄像头上的按键事件需要使用Android的USB Host API和UVC协议。
以下是一个简单的示例代码,用于读取UVC摄像头上的按键事件:
```java
public class UVCButtonActivity extends Activity implements View.OnClickListener {
private static final String TAG = "UVCButtonActivity";
private UsbDevice mDevice;
private UsbManager mUsbManager;
private UsbInterface mInterface;
private UsbEndpoint mEndpointIntr;
private boolean mIsReading = false;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.uvc_button_activity);
Button openButton = (Button) findViewById(R.id.open_button);
openButton.setOnClickListener(this);
Button closeButton = (Button) findViewById(R.id.close_button);
closeButton.setOnClickListener(this);
mUsbManager = (UsbManager) getSystemService(Context.USB_SERVICE);
}
@Override
protected void onResume() {
super.onResume();
if (mDevice == null) {
// Find the UVC camera device
for (UsbDevice device : mUsbManager.getDeviceList().values()) {
if (device.getVendorId() == UVC_VENDOR_ID && device.getProductId() == UVC_PRODUCT_ID) {
mDevice = device;
break;
}
}
if (mDevice == null) {
Log.e(TAG, "UVC camera device not found");
return;
}
// Find the UVC interface and endpoint
for (int i = 0; i < mDevice.getInterfaceCount(); i++) {
UsbInterface intf = mDevice.getInterface(i);
if (intf.getInterfaceClass() == UsbConstants.USB_CLASS_VIDEO && intf.getInterfaceSubclass() == UVC_SUBCLASS_CONTROL) {
mInterface = intf;
break;
}
}
if (mInterface == null) {
Log.e(TAG, "UVC camera interface not found");
return;
}
UsbEndpoint endpointIntr = null;
for (int i = 0; i < mInterface.getEndpointCount(); i++) {
UsbEndpoint endpoint = mInterface.getEndpoint(i);
if (endpoint.getType() == UsbConstants.USB_ENDPOINT_XFER_INT) {
endpointIntr = endpoint;
break;
}
}
if (endpointIntr == null) {
Log.e(TAG, "UVC camera endpoint not found");
return;
}
mEndpointIntr = endpointIntr;
}
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.open_button:
if (!mIsReading) {
mIsReading = true;
new Thread(new Runnable() {
@Override
public void run() {
readButtonEvent();
}
}).start();
}
break;
case R.id.close_button:
mIsReading = false;
break;
}
}
private void readButtonEvent() {
UsbDeviceConnection connection = mUsbManager.openDevice(mDevice);
if (connection == null) {
Log.e(TAG, "Failed to open UVC camera device");
return;
}
connection.claimInterface(mInterface, true);
byte[] buffer = new byte[4];
while (mIsReading) {
int result = connection.bulkTransfer(mEndpointIntr, buffer, buffer.length, 1000);
if (result >= 0) {
// Parse the button event
int buttonId = buffer[0];
int buttonState = buffer[1];
Log.i(TAG, "UVC button event: buttonId=" + buttonId + ", buttonState=" + buttonState);
}
}
connection.releaseInterface(mInterface);
connection.close();
}
}
```
需要注意的是,这只是一个简单的示例代码,实际情况可能需要根据具体的UVC摄像头硬件和协议进行修改。
阅读全文
相关推荐


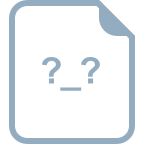
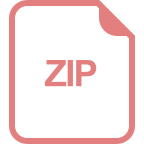



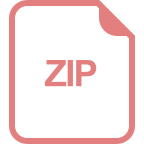
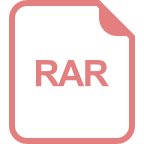






