//#include<iostream> //#include<vector> //using namespace std; // //int main() //{ // // 窗口坐标 x, y // vector<int> xn; // vector<int> yn; // // // 鼠标点击坐标 // vector<int> x; // vector<int> y; // // // 记录窗口顺序 // vector<int> s(10); // 使用 vector 代替动态数组 // // int win = -1; // int m = 0, n = 0, a = 0, b = 0, c = 0, d = 0, temp = 0; // cin >> n >> m; // // // 记录窗口信息 // for (int i = 0; i < n; i++) { // cin >> a >> b >> c >> d; // xn.push_back(a); // yn.push_back(b); // xn.push_back(c); // yn.push_back(d); // s[i] = i + 1; // } // // // 记录鼠标 // for (int i = 0; i < m; i++) { // cin >> a >> b; // x.push_back(a); // y.push_back(b); // } // // // 判断置顶窗口 // for (int i = 0; i < m; i++) { // // 遍历窗口位置 // for (int j = 0; j < n * 2; j += 2) { // // 找到顺序在最上面的窗口号 // if (xn[j] <= x[i] && x[i] <= xn[j + 1] && yn[j] <= y[i] && y[i] <= yn[j + 1]) { // if (j == 0) // win = s[j]; // else { // if (win == -1 || s[win - 1] < s[j - 1]) { // win = s[j - 1]; // } // } // } // } // // // 如果没找到 // if (win == -1) { // cout << "IGNORED" << endl; // continue; // } // // cout << win << endl; // // // 调整窗口顺序 // if (temp == win || i == 0) { // for (int k = 0; k < n; k++) { // if (s[k] > win) // s[k]--; // } // s[win] = n-1; // } // temp = win; // win = -1; // // } // // return 0; //}
时间: 2023-09-21 19:06:36 浏览: 38
这段代码实现了一个简单的窗口管理器。它接受输入来描述窗口和鼠标点击的坐标,并根据鼠标点击的位置确定需要置顶的窗口,并输出置顶窗口的编号。
该程序的实现逻辑如下:
1. 首先,从输入中读取 n 和 m,分别表示窗口数量和鼠标点击数量。
2. 然后,使用循环读取每个窗口的坐标信息,并将它们存储在 `xn` 和 `yn` 向量中,同时初始化窗口顺序向量 `s`。
3. 接下来,使用循环读取每个鼠标点击的坐标,并将它们存储在 `x` 和 `y` 向量中。
4. 针对每个鼠标点击的坐标,使用嵌套循环遍历窗口位置,找到包含该坐标的最上层窗口。
5. 如果找到了置顶窗口,则输出窗口编号并进行窗口顺序调整;否则,输出 "IGNORED"。
6. 在窗口顺序调整时,通过修改 `s` 向量来更新窗口顺序,将被置顶的窗口放在最上面,并递减其后面的窗口编号。
7. 最后,返回 0 表示程序正常结束。
请问有什么问题我可以帮助您解答吗?
相关问题
#include <iostream> #include <algorithm> #include <vector> using namespace std; int main() { int n; cin >> n; vector<int> nums(n); for (int i = 0; i < n; i++) { cin >> nums[i]; } sort(nums.begin(), nums.end()); // 排序
您的代码漏掉了大括号,请在sort语句后面加上大括号。另外,您需要加上输出结果的语句。完整的代码如下:
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
int main() {
int n;
cin >> n;
vector<int> nums(n);
for (int i = 0; i < n; i++) {
cin >> nums[i];
}
sort(nums.begin(), nums.end()); // 排序
for (int i = 0; i < n; i++) {
cout << nums[i] << " ";
}
return 0;
}
详细写出#include<iostream> #include<vector> using namespace std; vector<int> x,x1;//定义动态数组 vector<vector<int> > p; int powermax = -1; int n; //判断角色k的位置是否可行 bool position(int k){ for(int i = 1;i < k;i ++){ if(x[k] == x[i]){ return false; } } return true; } //回
答你的问题之前,我需要说明一下,我是一个语言模型AI,虽然我可以理解你的代码,但是我无法运行或测试它。不过我可以帮你解释一下你提供的代码段,希望能对你有所帮助。
这段代码主要涉及到动态数组和回溯算法。下面是对代码的解释:
```c++
#include<iostream>
#include<vector>
using namespace std;
vector<int> x, x1; //定义两个动态数组
vector<vector<int>> p; //定义一个二维动态数组
int powermax = -1; //初始化一个变量
int n;
//判断角色k的位置是否可行
bool position(int k){
for(int i = 1; i < k; i++){
if(x[k] == x[i]){
return false;
}
}
return true;
}
//回溯算法
void backtrack(int t){
if(t > n){ //当t>n时,说明已经完成了一组解,更新powermax的值
int power = 0;
for(int i = 1; i <= n; i++){
power += p[i][x1[i]];
}
powermax = max(powermax, power);
return;
}
for(int i = 1; i <= n; i++){ //枚举角色k的位置
x[t] = i;
if(position(t)){ //如果位置可行,继续往下递归
x1[i] = t;
backtrack(t + 1);
x1[i] = 0;
}
}
}
int main(){
cin >> n; //读入n的值
p.resize(n + 1); //调整p的大小
x.resize(n + 1); //调整x的大小
x1.resize(n + 1); //调整x1的大小
for(int i = 1; i <= n; i++){
p[i].resize(n + 1);
for(int j = 1; j <= n; j++){
cin >> p[i][j]; //读入p[i][j]的值
}
}
backtrack(1); //调用回溯算法
cout << powermax << endl; //输出结果
return 0;
}
```
这段代码实现了一个求解角色战斗力最大值的问题。其中,p[i][j]表示第i个角色在第j个位置上的战斗力值,x[i]表示第i个角色所在的位置,x1[i]表示第i个位置上的角色编号。
回溯算法是一种通过不断试错来寻找问题解法的算法。在这个例子中,回溯算法的主要思路是枚举每个角色的位置,然后判断该位置是否可行,如果可行,就继续往下递归,直到找到一组解或者发现当前位置不可行。如果找到一组解,就计算该解的战斗力值并与当前的最大值比较,更新最大值。
希望这些解释能帮助你更好地理解这段代码。如果你有其他问题或需要进一步的解释,欢迎随时问我。
相关推荐
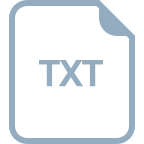
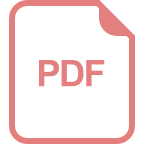
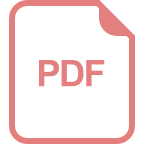













