用C语言编写连接到OPCUA服务器的代码
时间: 2024-10-09 19:12:54 浏览: 34
在C语言中,要连接到OPCUA服务器,你需要使用OPCUA的客户端库,比如Open62541(OpcUaClient)或其他的第三方库。以下是一个简单的示例,展示如何使用Open62541库连接到OPCUA服务器:
首先,请确保已安装了Open62541库。你可以在这里找到官方文档和下载链接:https://opcfoundation.org/opcua/
```c
#include < opcua.h> // 包含必要的头文件
// OPC UA服务器地址和端口
const char* server_url = "opc.tcp://localhost:4840";
UA_Url endpoint_url;
// 初始化OPC UA客户端
void init_opcua_client() {
if (UA_ClientCreate(&client, NULL) != UA_STATUSCODE_GOOD) {
printf("Error initializing client\n");
return;
}
// 解析服务器URL
UA_InitializeEndpointUrl(endpoint_url, server_url);
// 设置超时
UA_InitializeTimeouts(client, 1000); // 单位为毫秒
}
// 连接到OPC UA服务器
void connect_to_server(UA_Client *client) {
if (UA_ClientConnect(client, &endpoint_url, NULL) != UA_STATUSCODE_GOOD) {
printf("Failed to connect to server: %s\n", UA_STATUS_CODE_TO_STRING(UA_ClientGetLastSessionError(client)));
return;
}
printf("Connected to OPC UA server at %s\n", server_url);
}
// 关闭连接并释放资源
void close_opcua_connection(UA_Client *client) {
UA_ClientShutdown(client);
UA_ClientDelete(client);
}
int main() {
init_opcua_client();
// 连接到服务器
connect_to_server(client);
// 在这里执行你的OPC UA操作,例如读写节点、发布/订阅等
// 当完成操作后,关闭连接
close_opcua_connection(client);
return 0;
}
```
在这个示例中,`init_opcua_client()`初始化客户端,`connect_to_server(client)`尝试连接到指定的服务器,`close_opcua_connection(client)`用于关闭连接并清理资源。
请注意,这只是一个基础框架,实际应用中你可能还需要处理认证、错误处理、节点路径解析、以及根据具体需求执行相应的数据访问操作。
阅读全文
相关推荐
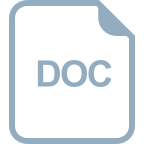
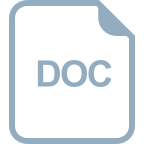
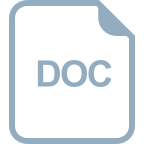
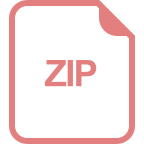

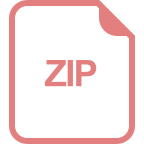
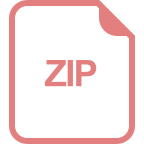
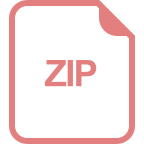
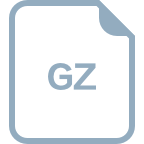
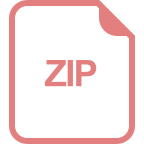
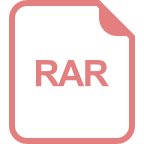
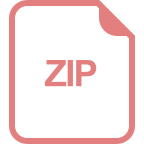



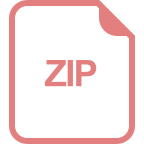
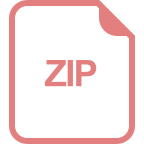

