用Python编写一个猫狗识别程序
时间: 2023-11-16 09:22:34 浏览: 116
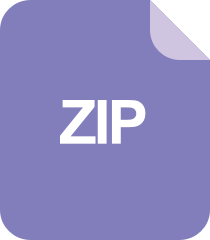
基于Python CNN的猫狗识别 web 应用【100012236】

好的,下面是一个简单的猫狗识别程序的实现过程:
1. 数据准备
首先,需要准备用于训练和测试的猫狗图片数据集。可以在网上找到一些现成的数据集,例如Kaggle的Dogs vs. Cats数据集。
2. 特征提取
接下来,需要对图片进行特征提取。可以使用预训练的卷积神经网络(CNN)模型来提取图片的特征向量。这里可以使用Keras框架中的VGG16模型。
3. 模型训练
使用提取的特征向量作为输入,训练一个分类器模型,例如使用支持向量机(SVM)或随机森林(Random Forest)等分类器。这里可以使用Scikit-learn库。
4. 模型评估
使用测试集来评估模型的性能,计算准确率、召回率、F1值等指标。
5. 预测
最后,使用训练好的模型来预测新的猫狗图片的类别。
下面是实现代码的一个简单示例:
```
# 导入必要的库
from keras.applications.vgg16 import VGG16
from keras.preprocessing import image
from keras.applications.vgg16 import preprocess_input
import numpy as np
import os
from sklearn.svm import SVC
from sklearn.metrics import accuracy_score, classification_report
from sklearn.model_selection import train_test_split
# 加载预训练的VGG16模型
model = VGG16(weights='imagenet', include_top=False)
# 定义函数来提取图片特征向量
def extract_features(img_path):
img = image.load_img(img_path, target_size=(224, 224))
x = image.img_to_array(img)
x = np.expand_dims(x, axis=0)
x = preprocess_input(x)
features = model.predict(x)
return features.flatten()
# 加载数据集,提取特征向量并标记类别
dataset_path = '/path/to/dataset'
features = []
labels = []
for root, dirs, files in os.walk(dataset_path):
for file in files:
if file.endswith('.jpg'):
img_path = os.path.join(root, file)
feature = extract_features(img_path)
features.append(feature)
if 'cat' in file:
labels.append(0)
elif 'dog' in file:
labels.append(1)
# 划分训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(features, labels, test_size=0.2, random_state=42)
# 训练一个SVM分类器
clf = SVC(kernel='linear')
clf.fit(X_train, y_train)
# 在测试集上评估模型
y_pred = clf.predict(X_test)
print('Accuracy:', accuracy_score(y_test, y_pred))
print(classification_report(y_test, y_pred))
# 使用模型预测新的猫狗图片
new_img_path = '/path/to/new/image'
new_feature = extract_features(new_img_path)
new_label = clf.predict([new_feature])
if new_label == 0:
print('This is a cat.')
else:
print('This is a dog.')
```
注意事项:
- 在实际应用中,可能需要对数据集进行进一步的处理和增强,例如数据增强、数据平衡等。
- 对于大规模数据集,可能需要使用分布式计算框架来进行模型的训练和评估。
阅读全文
相关推荐
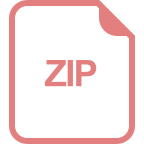
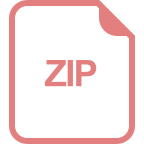
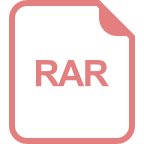
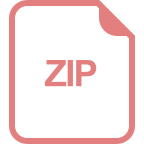
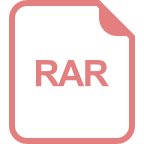
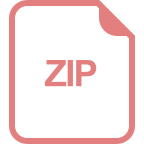
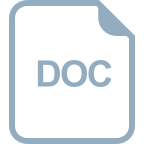
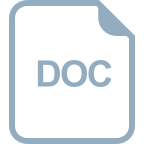
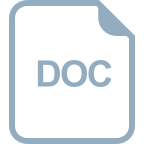
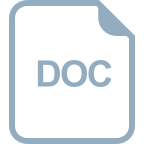







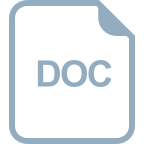