实现函数int dup(char *str)函数名:dup 功能:将数组str[]中的小写字母变成大写字母,并把其中的数字去掉如: Hello2010Word2011 !HELLOWORD!) 参数: str[]字符串数组 返回值,返回去掉了多少个数字
时间: 2024-03-06 09:47:04 浏览: 64
下面是实现函数int dup(char *str)的代码:
```
int dup(char *str) {
int i, j = 0, count = 0;
for (i = 0; str[i] != '\0'; i++) {
if (str[i] >= 'a' && str[i] <= 'z') {
str[j++] = str[i] - 'a' + 'A'; // 小写字母变成大写字母
} else if (str[i] < '0' || str[i] > '9') {
str[j++] = str[i]; // 非数字直接复制
} else {
count++; // 数字计数
}
}
str[j] = '\0'; // 字符串结尾
return count;
}
```
函数中使用了两个指针,一个用于遍历原字符串,一个用于记录新字符串的位置。如果遇到小写字母,则将其变成大写字母并复制到新字符串中;如果遇到非数字,则直接复制到新字符串中;如果遇到数字,则计数器加一。最后在新字符串的末尾加上字符串结尾符号,并返回数字计数器的值。
相关问题
c++实现函数int dup(char *str)函数名: dup 功能:将数组str中的小写字母变成大写字母,并把其中的数字去掉如:Hello2010Word2011 !HELLOWORD!) 参数:str字符串数组 返回值,返回去掉了多少个数字
以下是C++实现的`dup`函数,用于将字符串数组中的小写字母变成大写字母,并去掉其中的数字。函数返回去掉了多少个数字。
```cpp
#include <iostream>
#include <cctype> // 包含字符处理函数toupper和isdigit的头文件
int dup(char *str) {
int count = 0; // 记录去掉的数字个数
int i = 0; // 用于遍历字符串数组的索引
while (str[i] != '\0') {
if (islower(str[i])) { // 判断是否为小写字母
str[i] = toupper(str[i]); // 将小写字母变为大写字母
} else if (isdigit(str[i])) { // 判断是否为数字
count++; // 去掉一个数字,计数器加一
int j = i; // 用于移动字符的索引
while (str[j] != '\0') {
str[j] = str[j+1]; // 将后续字符往前移动,覆盖掉当前的数字
j++;
}
continue; // 继续下一次循环,避免i自增
}
i++;
}
return count;
}
int main() {
char str[] = "Hello2010Word2011";
int removedDigits = dup(str);
std::cout << "Modified string: " << str << std::endl;
std::cout << "Removed digits count: " << removedDigits << std::endl;
return 0;
}
```
上述代码中,使用了`cctype`头文件中的`toupper`函数和`isdigit`函数进行字符处理。在遍历字符串数组时,判断字符是小写字母则转为大写字母,判断字符是数字则移除该数字并增加计数器的值。最后,输出修改后的字符串和去掉的数字个数。
注意:上述实现假设传入的字符串数组以空字符`\0`结尾,且数组长度足够容纳修改后的字符串。在实际使用时,需要根据实际情况进行适当的修改和处理。
实现函数int dup(char *str)函数名: dup 功能:将数组str[]中的小写字母变成大写字母,并把其中的数字去掉如:Hello2010Word2011 !HELLOWORD!) 参数: str[]字符串数组 返回值,返回去掉了多少个数字
以下是实现函数int dup(char *str)的代码:
```c
#include <stdio.h>
#include <ctype.h>
int dup(char *str) {
int count = 0;
int i, j;
for (i = 0, j = 0; str[i] != '\0'; i++) {
if (isdigit(str[i])) {
count++;
} else if (islower(str[i])) {
str[j++] = toupper(str[i]);
} else if (isupper(str[i])) {
str[j++] = str[i];
}
}
str[j] = '\0';
return count;
}
int main() {
char str[] = "Hello2010Word2011";
int count = dup(str);
printf("%s\n", str);
printf("Removed %d digits.\n", count);
return 0;
}
```
在dup函数中,我们遍历输入的字符串数组,对于每个字符,如果它是小写字母,我们将其转换成大写字母并添加到输出的新字符串数组中;如果它是大写字母,我们直接添加到新字符串数组中;如果它是数字,我们将计数器count加1。最后,我们将新字符串数组的末尾设置为null字符,以便printf函数能正确输出新字符串。最后,我们在主函数中调用dup函数,并输出处理后的新字符串和删除的数字数。
阅读全文
相关推荐
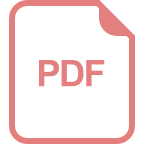
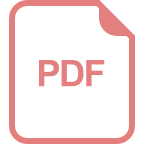












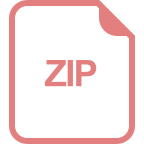