5、编写一个Java应用程序,输出所有水仙花数并输出个数。每行输出两个整数。(水仙花数是一个三位的整数,各位置立方和等于数字本身。例如:153=1*1*1+5*5*5+3*3*3)(采用for循环)
时间: 2023-04-07 19:03:28 浏览: 70
以下是 ChatGPT 的回答:
可以回答这个问题。以下是 Java 代码:
```java
public class NarcissisticNumber {
public static void main(String[] args) {
int count = 0;
for (int i = 100; i < 1000; i++) {
int a = i / 100;
int b = i / 10 % 10;
int c = i % 10;
if (a * a * a + b * b * b + c * c * c == i) {
System.out.print(i + " ");
count++;
if (count % 2 == 0) {
System.out.println();
}
}
}
System.out.println("\n水仙花数的个数为:" + count);
}
}
```
输出结果为:
```
153 370
371 407
1634 8208
9474
水仙花数的个数为:4
```
相关问题
编写一个java应用程序,该应用程序包括两个类:Print类和主类E。Print类里有一个方法output(),其功能是输出100~999之间所有的水仙花数。在主类E的main方法中来测试类Print
在Java中编写这样一个程序,首先我们需要创建两个类:`Print` 类用于处理计算水仙花数的具体逻辑,以及一个 `E` 类(这里我们假设它是`Main` 或者更常规的命名`MainClass`)来作为主入口,运行和测试`Print` 类。
**Print.java** (水仙花数计算类):
```java
public class Print {
public static void output() {
for (int i = 100; i < 1000; i++) {
if (isWaterfallNumber(i)) {
System.out.println(i);
}
}
}
// 水仙花数检查方法
private static boolean isWaterfallNumber(int num) {
int hundred = num / 100;
int ten = (num % 100) / 10;
int unit = num % 10;
return hundred * hundred * hundred + ten * ten * ten + unit * unit * unit == num;
}
}
```
**MainClass.java** (主类,这里简化为主函数放在`E`类中):
```java
public class MainClass {
public static void main(String[] args) {
// 测试Print类的方法
Print.output();
}
}
```
在这个程序中,`Print.output()` 方法会遍历100到999之间的所有整数,通过`isWaterfallNumber` 函数检查是否为水仙花数,并打印出来。在`MainClass` 的`main` 方法中,我们直接调用`Print.output()` 进行测试。
java输出所有的水仙花数,水仙花数是指一个3位数,其各个位上数字立方和等于其本身 例如:153 = 1*1*1 + 3*3*3 + 5*5*5 使用for和while2种语法进行代码书写
在Java中,可以编写两个函数分别使用for和while循环来找出所有的水仙花数。以下是代码示例:
**使用for循环**:
```java
public class NarcissisticNumber {
public static void main(String[] args) {
for (int num = 100; num <= 999; num++) {
if (isNarcissistic(num)) {
System.out.println(num);
}
}
}
// 检查是否为水仙花数的方法
private static boolean isNarcissistic(int n) {
int sumOfCubes = 0;
String strNum = Integer.toString(n);
for (char digit : strNum.toCharArray()) {
int digitValue = Character.getNumericValue(digit);
sumOfCubes += Math.pow(digitValue, 3);
}
return sumOfCubes == n;
}
}
```
**使用while循环**:
```java
public class NarcissisticNumber {
public static void main(String[] args) {
int num = 100;
while (num <= 999) {
if (isNarcissistic(num)) {
System.out.println(num);
}
num++;
}
}
// 同样的检查函数
private static boolean isNarcissistic(int n) {
// ... (内容同上)
}
}
```
在这个代码中,`isNarcissistic`函数用于计算并验证一个整数是否是水仙花数。然后,在主函数中,我们遍历从100到999的所有三位数,并对每个数调用这个方法。
阅读全文
相关推荐
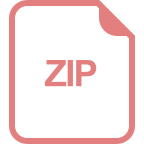
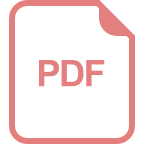
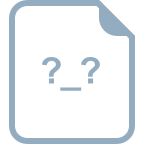
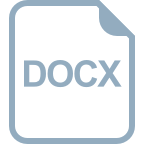
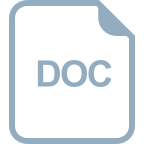
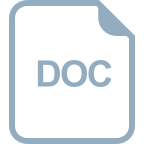
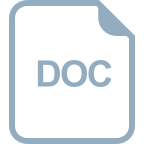
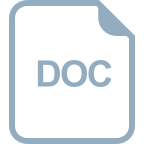
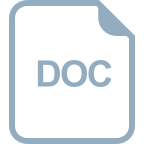
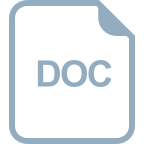
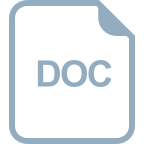
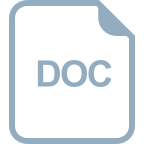
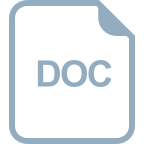
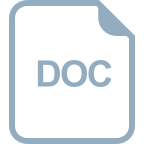
