s=Student(name=name,age=age,sex = sex,hobby=hobby,birthday=birthday) 为什么name=name 这样表示
时间: 2024-11-08 21:17:59 浏览: 11
在这个例子中,`s=Student(name=name,age=age,sex = sex,hobby=hobby,birthday=birthday)` 的写法看起来有些不寻常。实际上,这并不是标准的JavaScript或其他面向对象编程语言的语法。它更像是一个简化的示意,意图表达的是使用某种方式为 `Student` 类的实例 `s` 设置初始属性,其中每个属性都等于对应的变量名。
如果这是一个函数调用,可能是为了从外部传入一组关键字参数(key-value pairs),并将它们作为默认值传递给 `Student` 构造函数。例如,如果 `name`, `age`, `sex`, `hobby`, 和 `birthday` 已经预先定义了对应值,那么这样做是为了在创建 `Student` 实例时自动应用这些值。
然而,在实际代码中,通常你会这样写:
```javascript
var s = new Student('John Doe', 20, 'Male', 'Reading', '1990-01-01');
```
或者是这样的:
```python
s = Student(name='John', age=20, sex='Male', hobby='Reading', birthday='1990-01-01')
```
这两个示例都是明确地为 `s` 创建一个新的 `Student` 对象,并赋予特定的属性值。至于 `name=name` 这样的写法,如果不是特殊上下文或代码片段的一部分,就不太常见,也不建议在正式代码中使用。
相关问题
package form; public class Student { private String id; //学号 private String name; //姓名 private String sex; //性别 private String age; //年龄 private String birthday; //出生日期 private String college; //学院 private int grade; //成绩 //将所有的成员变量都设置成为private类型,使用setter和getter对成员变量进行赋值 public Student() { } public Student(String id, String name, String sex, String age, String birthday, String college, int grade) { this.id = id; this.name = name; this.sex = sex; this.age = age; this.birthday = birthday; this.college = college; this.grade = grade; }//student集合 public String getId() { return id; } public void setId(String id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getSex() { return sex; } public void setSex(String sex) { this.sex = sex; } public String getAge() { return age; } public void setAge(String age) { this.age = age; } public String getBirthday() { return birthday; } public void setBirthday(String birthday) { this.birthday = birthday; } public String getCollege() { return college; } public void setCollege(String college) { this.college = college; } public int getGrade() { return grade; } public void setGrade(int grade) { this.grade = grade; } }
这段代码是一个Java类,名为Student,用于表示一个学生的信息。它有私有成员变量id、name、sex、age、birthday、college和grade,分别表示学号、姓名、性别、年龄、出生日期、学院和成绩。这些成员变量都被设置为private类型,因此只能通过公有的setter和getter方法对它们进行赋值和访问。构造函数有一个无参构造函数和一个带有七个参数的构造函数,用于创建Student对象并初始化其成员变量。
①创建Student类,Student类中有name姓名,sex性别,age年龄,hobby外号4个属性,根据属性含义定义它们的属性类型。
当然可以,我们创建一个名为`Student`的基本Java类,它包含四个私有成员变量分别表示姓名、性别、年龄和爱好。下面是这个类的定义:
```java
public class Student {
// 定义属性并设置其数据类型
private String name; // 姓名(字符串类型)
private char sex; // 性别(字符类型,通常用大写字母'M'或'F'表示男性或女性)
private int age; // 年龄(整数类型)
private String hobby; // 外号(字符串类型)
// 构造函数用于初始化这些属性
public Student(String name, char sex, int age, String hobby) {
this.name = name;
this.sex = sex;
this.age = age;
this.hobby = hobby;
}
// getter和setter方法用于获取和设置属性值
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public char getSex() {
return sex;
}
public void setSex(char sex) {
this.sex = sex;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getHobby() {
return hobby;
}
public void setHobby(String hobby) {
this.hobby = hobby;
}
}
```
在这个类中,通过getter和setter方法我们可以安全地访问和修改这些私有属性。
阅读全文
相关推荐
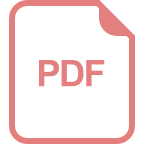
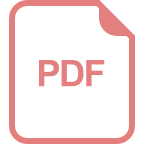
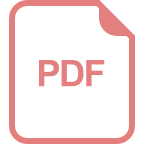
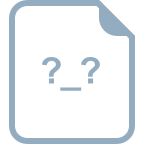
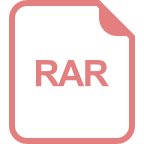
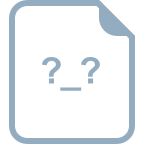
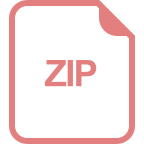
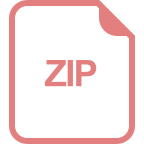
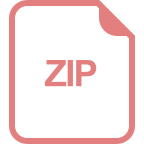
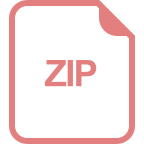
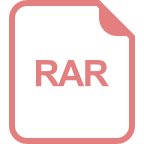
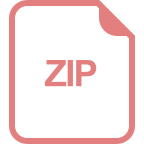





