对smote算法完成以下的改进并给出详细的python代码以及应用实例。合成时不只是考虑原始的数据样本,也要考虑合成后的数据样本如何影响全局数据,可以考虑在每次合成数据之后将其加入到数据集,在处理过程中将合成数据也加入考虑范围。
时间: 2024-02-20 17:01:25 浏览: 22
在SMOTE算法中,合成新样本时,通常只考虑原始数据样本之间的相似性,而忽略了合成后的数据样本对于整个数据集的影响。为了更好地考虑全局数据的影响,我们可以在每次合成数据之后,将其加入到数据集中,然后重新计算最近邻的距离,并继续进行合成,直到满足指定的合成数据比例。这样做的好处是,每次合成数据时,都会考虑到新的合成数据对于整个数据集的影响,从而更好地保持数据分布的一致性。
下面给出一个基于这个思路的改进版SMOTE算法的Python实现。首先,我们需要定义一个函数来计算每个样本的最近邻距离:
```python
from sklearn.neighbors import NearestNeighbors
def calculate_nearest_neighbors(data, k):
knn = NearestNeighbors(n_neighbors=k+1).fit(data)
distances, indices = knn.kneighbors(data)
return distances[:, 1:], indices[:, 1:]
```
然后,我们可以定义一个函数来实现改进版的SMOTE算法:
```python
import numpy as np
def SMOTE_improved(X, y, k=5, ratio=1.0, n_iterations=5):
# k: number of nearest neighbors to consider
# ratio: target ratio of synthetic samples to real samples
# n_iterations: number of iterations to perform
# Determine the minority class samples
minority_class = np.min(y)
X_minority = X[y==minority_class]
# Determine the number of synthetic samples to generate
n_synthetic = int(ratio * len(X_minority) - len(X_minority))
# Create synthetic samples
if n_synthetic > 0:
# Perform multiple iterations to consider the global data distribution
for i in range(n_iterations):
# Compute the nearest neighbors for each sample
distances, indices = calculate_nearest_neighbors(X, k)
# Generate synthetic samples
synthetic_samples = []
for j in range(len(X_minority)):
# Select a random minority class sample
idx = np.random.randint(len(X_minority))
sample = X_minority[idx]
# Compute the importance weights for the k nearest neighbors
weights = np.ones(k)
for l in range(k):
neighbor = indices[idx][l]
if y[neighbor] == minority_class:
weights[l] = 1.0
else:
# Compute the distance between the sample and the neighbor
distance = distances[idx][l]
# Compute the inverse distance as the weight
weights[l] = 1.0 / distance
weights /= weights.sum()
# Generate a synthetic sample as a weighted sum of the k nearest neighbors
synthetic_sample = np.zeros_like(sample)
for l in range(k):
neighbor = indices[idx][l]
weight = weights[l]
synthetic_sample += weight * (X[neighbor] - sample)
synthetic_samples.append(sample + synthetic_sample)
# Add the synthetic samples to the data set
X = np.vstack((X, np.array(synthetic_samples)))
y = np.hstack((y, np.array([minority_class]*n_synthetic)))
return X, y
```
这个改进版的SMOTE算法与原始的SMOTE算法相比,只是在每次合成数据之后,将新的合成数据加入到数据集中,并重新计算最近邻距离。这样做可以使得合成数据更好地考虑到全局数据的影响,从而更好地保持数据分布的一致性。
下面给出一个简单的应用实例,来演示如何使用改进版的SMOTE算法来处理不平衡数据集:
```python
from sklearn.datasets import make_classification
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import classification_report
# Generate an imbalanced data set
X, y = make_classification(n_classes=2, class_sep=2, weights=[0.1, 0.9], n_informative=3, n_redundant=1, flip_y=0, n_features=20, n_clusters_per_class=1, n_samples=1000, random_state=10)
# Split the data set into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=10)
# Apply SMOTE to the training set
X_train_resampled, y_train_resampled = SMOTE_improved(X_train, y_train, k=5, ratio=1.0, n_iterations=5)
# Train a logistic regression model on the resampled data set
clf = LogisticRegression()
clf.fit(X_train_resampled, y_train_resampled)
# Evaluate the model on the testing set
y_pred = clf.predict(X_test)
print(classification_report(y_test, y_pred))
```
在这个应用实例中,我们使用了`make_classification`函数生成一个不平衡的二分类数据集,然后使用改进版的SMOTE算法对训练集进行过采样,最后使用逻辑回归模型对采样后的数据集进行训练并在测试集上进行评估。
相关推荐
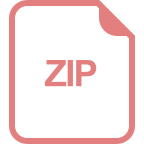
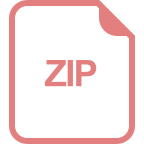














