springboot如何通过caffeine和@Cacheable实现定时刷新
时间: 2023-08-11 15:04:10 浏览: 749
要 Caffeine 和 `@Cacheable` 注解实现定时刷新缓存,可以按照以下步骤进行操作:
1. 添加 Caffeine 依赖:在项目的 `pom.xml` 文件中添加 Caffeine 依赖。
```xml
<dependency>
<groupId>com.github.ben-manes.caffeine</groupId>
<artifactId>caffeine</artifactId>
<version>2.9.0</version>
</dependency>
```
2. 创建缓存配置:在 Spring Boot 的配置类中创建一个缓存配置类,配置 Caffeine 缓存。
```java
import com.github.benmanes.caffeine.cache.Caffeine;
import org.springframework.cache.CacheManager;
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.cache.caffeine.CaffeineCacheManager;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import java.util.concurrent.TimeUnit;
@Configuration
@EnableCaching
public class CacheConfig {
@Bean
public CacheManager cacheManager() {
CaffeineCacheManager cacheManager = new CaffeineCacheManager("myCache");
cacheManager.setCaffeine(caffeineCacheBuilder());
return cacheManager;
}
Caffeine<Object, Object> caffeineCacheBuilder() {
return Caffeine.newBuilder()
.expireAfterWrite(1, TimeUnit.HOURS) // 缓存项写入后过期时间
.refreshAfterWrite(30, TimeUnit.MINUTES) // 缓存项刷新时间
.maximumSize(100) // 缓存最大大小
.weakKeys() // 弱引用键
.recordStats(); // 记录统计信息
}
}
```
3. 使用 `@Cacheable` 注解:在需要进行缓存的方法上使用 `@Cacheable` 注解,并指定缓存的名称。
```java
import org.springframework.cache.annotation.Cacheable;
import org.springframework.stereotype.Service;
@Service
public class MyService {
@Cacheable(cacheNames = "myCache")
public String getData(String key) {
// 从数据库或其他数据源获取数据
return "data";
}
}
```
4. 定时刷新缓存:使用 Spring 的定时任务功能,定时调用需要刷新缓存的方法。
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.cache.CacheManager;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class CacheScheduler {
private final CacheManager cacheManager;
private final MyService myService;
@Autowired
public CacheScheduler(CacheManager cacheManager, MyService myService) {
this.cacheManager = cacheManager;
this.myService = myService;
}
@Scheduled(fixedRate = 30 * 60 * 1000) // 每30分钟执行一次
public void refreshCache() {
cacheManager.getCache("myCache").clear();
myService.getData("key"); // 触发缓存的重新加载
}
}
```
以上步骤将使用 Caffeine 缓存和 `@Cacheable` 注解实现缓存的定时刷新。在每次定时任务执行时,会清空缓存并重新加载数据。请根据自己的需求进行适当调整和配置。
阅读全文
相关推荐
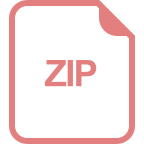
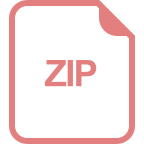






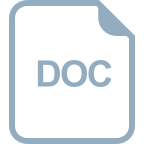








