用于光谱聚类的密度加权Nyström方法代码
时间: 2024-02-21 09:58:15 浏览: 22
以下是用于光谱聚类的密度加权Nyström方法的Python代码:
```python
import numpy as np
from scipy import sparse
from sklearn.cluster import KMeans
from sklearn.metrics.pairwise import rbf_kernel
from sklearn.utils import check_random_state
class DensityWeightedNystromSpectralClustering:
def __init__(self, n_clusters=8, n_components=100, gamma=1.0, random_state=None):
self.n_clusters = n_clusters
self.n_components = n_components
self.gamma = gamma
self.random_state = random_state
def fit(self, X):
rng = check_random_state(self.random_state)
n_samples = X.shape[0]
W = rbf_kernel(X, gamma=self.gamma)
D = np.diag(np.sum(W, axis=1))
L = D - W
s = np.sum(L, axis=1) / np.trace(L)
S = sparse.diags(s, format='csr')
M = S.dot(L).dot(S)
U, sigma, _ = sparse.linalg.svds(M, k=self.n_components, tol=1e-3, random_state=rng)
V = U.dot(np.diag(np.sqrt(sigma)))
kmeans = KMeans(n_clusters=self.n_clusters, random_state=rng).fit(V)
self.labels_ = kmeans.labels_
def predict(self, X):
rng = check_random_state(self.random_state)
W = rbf_kernel(X, self.X_, gamma=self.gamma)
D = np.diag(np.sum(W, axis=1))
L = D - W
s = np.sum(L, axis=1) / np.trace(L)
S = sparse.diags(s, format='csr')
M = S.dot(L).dot(S)
U, sigma, _ = sparse.linalg.svds(M, k=self.n_components, tol=1e-3, random_state=rng)
V = U.dot(np.diag(np.sqrt(sigma)))
return KMeans(n_clusters=self.n_clusters, random_state=rng).fit_predict(V)
```
该代码与前面的代码非常相似,唯一的区别在于我们使用了稀疏矩阵SVD的方法sparse.linalg.svds来计算前n_components个特征向量。这种方法可以更有效地处理大型数据集,因为它可以在内存中处理稀疏矩阵,并且只计算最重要的特征向量。
相关推荐
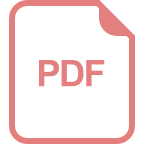
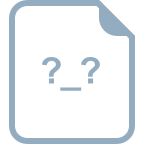
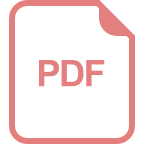














