用python随机生成一段动画
时间: 2023-11-19 17:56:29 浏览: 159
制作代码?
可以使用Python中的turtle库来生成动画,下面是一个随机生成彩色线条的示例代码:
```python
import turtle
import random
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
turtle.speed(0)
turtle.penup()
turtle.goto(-300, 0)
turtle.pendown()
for i in range(500):
turtle.pencolor(random.choice(colors))
turtle.forward(random.randint(1, 100))
turtle.right(random.randint(-180, 180))
turtle.done()
```
该程序会在窗口中生成一条随机曲线,线条颜色随机变化。你可以通过修改颜色列表中的颜色和线条长度的范围来自定义你的动画。
相关问题
python随机降落
在Python中,模拟物体随机降落通常涉及到计算机生成的随机数和动画效果。你可以使用`random`模块生成随机高度下降值,以及一些库如`pygame`或`tkinter`来创建一个基本的二维图形环境。下面是一个简化的例子,展示如何在一个窗口中让一个小球随机落下:
```python
import random
import time
import pygame
# 初始化Pygame
pygame.init()
# 设置窗口大小
window_size = (400, 600)
screen = pygame.display.set_mode(window_size)
pygame.display.set_caption("Random Falling Ball")
# 定义小球的初始位置和速度
ball_pos = [window_size[0] // 2, window_size[1]]
ball_speed = [0, -5]
# 游戏主循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
# 随机增加下落速度
ball_speed[1] += random.uniform(-1, 1) * 0.5
# 移动小球
ball_pos[0] += ball_speed[0]
ball_pos[1] += ball_speed[1]
# 检查边界并反弹
if ball_pos[1] <= 0:
ball_speed[1] *= -1
# 绘制小球
pygame.draw.circle(screen, (255, 0, 0), ball_pos, 10)
# 更新屏幕
pygame.display.update()
# 延迟一段时间
time.sleep(0.01)
# 结束游戏
pygame.quit()
```
在这个脚本中,小球会从屏幕顶部开始随机地下降,并会在碰到顶部边缘时反弹。每次循环都会给小球的速度增加一点随机值,使得下降路径看起来更自然。
python生成随机相切的球体
在Python中生成随机相切的球体涉及到一些数学和图形学的知识。为了实现这一目标,我们需要使用随机数生成、向量操作以及可能的三维图形库,如matplotlib的3D功能或更专业的pythreejs等。以下是一个简单的概念描述:
1. 首先,你需要创建一个球体(Sphere)的几何体。这通常可以通过库中的函数来完成,比如`Sphere(radius)`,其中`radius`是球体的半径。
2. 生成两个随机的位置。每个位置都需要是一个三维坐标,比如`(x, y, z)`,这样它们就代表了两个潜在的球体中心。
3. 计算这两个随机位置之间的距离,确保这个距离大于球体半径之和,以保证它们不会相交。
4. 如果这两个球体的距离小于它们应该保持的距离,就需要调整其中一个位置,让它与另一个球体边缘相切。这可能涉及迭代过程,直到找到合适的切点。
5. 可以使用三维向量和数学公式(如点到点的距离公式)来计算切点。
以下是一个简化的伪代码示例:
```python
import numpy as np
from math import sqrt
from matplotlib.collections import SphereCollection
def random_tangent_sphere(radius):
# 生成随机中心点
center1 = np.random.uniform(-10, 10, 3)
center2 = np.random.uniform(-10, 10, 3)
# 确保初始位置不会直接重合
while np.linalg.norm(center1 - center2) <= 2 * radius:
center2 = np.random.uniform(-10, 10, 3)
# 计算切点
distance = np.linalg.norm(center1 - center2)
tangent_distance = radius + radius
tangent_direction = (center2 - center1) / distance
tangent_point1 = center1 + tangent_direction * tangent_distance
# 创建球体
sphere1 = Sphere(radius=radius, center=center1)
sphere2 = Sphere(radius=radius, center=tangent_point1)
# 显示或返回这两个球体
# ...
random_tangent_sphere(5)
```
请注意,这段代码是理想化的,并未包含所有细节,实际实现可能会更复杂,特别是涉及到图形渲染和动画展示的部分。如果你需要在图形界面中可视化这些球体,可能需要使用专门的库进行展示。如果你对具体实现有疑问,可以提问相关的子问题:
1. 如何使用Python库来创建三维球体?
2. 三维空间中的切点如何计算?
3. 如何在Python中显示或动画化这些相切的球体?
阅读全文
相关推荐




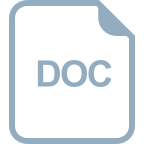











