有3个变量,x_range=[0, 10, 1], y_range=[0, 10, 1],z_range=[1.5, 1.6],以这3个变量为坐标轴组成一个网格,从网格上的随机一个点出发依次遍历网格上相邻的每个点,使用python编写代码绘制一段动画描述该过程
时间: 2023-08-31 21:35:15 浏览: 113
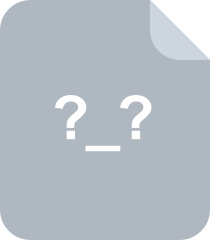
绘制关于x,y的三维图
### 回答1:
答:我可以使用Python编写代码来绘制一段动画来描述该过程,首先定义三个变量x,y,z分别表示三个轴的范围,然后使用网格函数生成网格坐标,从随机一个点出发,依次遍历网格上的每个点,在每一次遍历单元格之后,使用matplotlib库绘制出当前遍历过程,最后传入真实的数据生成动画,以描述这一过程。
### 回答2:
可以使用Python中的matplotlib和numpy库来编写代码绘制该过程的动画。首先,我们需要导入相应的库:
```python
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.animation as animation
```
然后,定义x_range、y_range和z_range:
```python
x_range = np.arange(0, 11, 1)
y_range = np.arange(0, 11, 1)
z_range = np.arange(1.5, 1.6, 0.1)
```
接下来,我们需要定义一个函数来生成所有相邻的点。当网格的一个点坐标为(x, y, z)时,相邻点的坐标为(x-1, y, z),(x+1, y, z),(x, y-1, z),(x, y+1, z),(x, y, z-0.1)和(x, y, z+0.1)。定义如下:
```python
def generate_adjacent_points(point):
adjacent_points = []
for i in [-1, 1]:
adjacent_points.append((point[0]+i, point[1], point[2]))
adjacent_points.append((point[0], point[1]+i, point[2]))
adjacent_points.append((point[0], point[1], point[2]-0.1))
adjacent_points.append((point[0], point[1], point[2]+0.1))
return adjacent_points
```
然后,定义一个函数用于绘制动画:
```python
def animate(i):
point = np.random.choice(x_range), np.random.choice(y_range), np.random.choice(z_range)
adjacent_points = generate_adjacent_points(point)
x = [point[0] for point in adjacent_points]
y = [point[1] for point in adjacent_points]
z = [point[2] for point in adjacent_points]
ax.clear()
ax.scatter(x, y, z, c='b')
ax.set_xlim(0, 11)
ax.set_ylim(0, 11)
ax.set_zlim(1.4, 1.7)
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ani = animation.FuncAnimation(fig, animate, interval=1000, frames=10)
plt.show()
```
最后,调用`animation.FuncAnimation`函数来生成动画,`interval`参数用于设置每一帧之间的间隔时间,`frames`参数用于设置动画的总帧数。
运行代码,即可看到一个描述从网格上随机一个点出发依次遍历相邻点的动画。
### 回答3:
首先,我们可以使用numpy中的arange函数生成x_range和y_range范围内的点的坐标:
```python
import numpy as np
x_range = np.arange(0, 11, 1)
y_range = np.arange(0, 11, 1)
z_range = np.arange(1.5, 1.6, 0.01)
```
然后,我们可以使用matplotlib库的animation模块绘制动画。具体步骤如下:
1. 首先,导入需要的库:
```python
import matplotlib.pyplot as plt
import matplotlib.animation as animation
```
2. 创建画布和子图:
```python
fig = plt.figure()
ax = plt.axes(projection='3d')
```
3. 定义初始状态和每一帧的更新函数:
```python
def init():
return []
def update(frame):
ax.clear()
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
ax.scatter(frame[0], frame[1], frame[2], color='red')
return ax.scatter(frame[0], frame[1], frame[2], color='red')
```
4. 遍历网格上的每个点,将每个点作为帧添加到动画中:
```python
frames = []
for x in x_range:
for y in y_range:
for z in z_range:
frames.append([x, y, z])
ani = animation.FuncAnimation(fig, update, frames=frames, init_func=init, blit=True)
```
5. 显示动画:
```python
plt.show()
```
综上所述,通过以上代码,我们可以使用python编写代码绘制一段动画,描述从网格上的随机一个点出发依次遍历网格上相邻的每个点的过程。
阅读全文
相关推荐
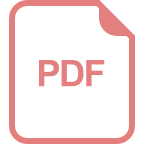
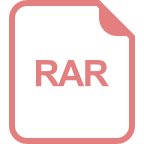












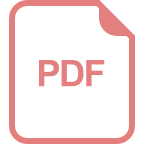
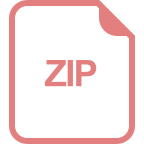
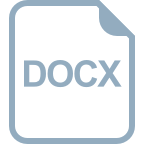