有3个变量,x_range=[0, 10, 1], y_range=[0, 10, 1],z_range=[1.5, 1.6],以这3个变量为坐标轴组成一个网格,从网格上的随机一个点出发依次遍历网格上相邻的每个点,给出代码,使用python编写代码绘制一段动画描述该过程
时间: 2023-09-15 17:06:01 浏览: 70
### 回答1:
import matplotlib.pyplot as plt
import numpy as np
x_range = [0, 10, 1]
y_range = [0, 10, 1]
z_range = [1.5, 1.6]# Generate grid points
x, y = np.meshgrid(np.arange(*x_range), np.arange(*y_range))# Generate random point
x_rand, y_rand = np.random.randint(*x_range), np.random.randint(*y_range)# Generate figure
fig, ax = plt.subplots()
ax.scatter(x, y, z_range[0], c='orange', label='Grid Points')
ax.scatter(x_rand, y_rand, z_range[1], c='b', label='Random Point')# Update figure
ax.plot([x_rand], [y_rand], color='b', linewidth=2, label='Path')
ax.legend(loc='upper center')# Traverse grid points
for i in np.arange(x_range[0], x_range[1], x_range[2]):
for j in np.arange(y_range[0], y_range[1], y_range[2]):
ax.plot([x_rand, i], [y_rand, j], color='b', linewidth=2)
x_rand, y_rand = i, j# Show figure
plt.show()
### 回答2:
请见以下Python代码,用于绘制一个描述网格上随机一个点出发依次遍历相邻点的动画:
```python
import numpy as np
import matplotlib.pyplot as plt
from matplotlib.animation import FuncAnimation
# 定义网格的范围
x_range = np.arange(0, 10, 1)
y_range = np.arange(0, 10, 1)
z_range = np.arange(1.5, 1.6, 0.1)
# 随机选择一个起始点
start_point = (np.random.choice(x_range), np.random.choice(y_range), np.random.choice(z_range))
current_point = start_point
# 定义绘制函数,用于在每一帧绘制图像
def update(frame):
global current_point
plt.cla() # 清除当前图形
# 绘制网格
plt.grid(True)
# 绘制所有点
for x in x_range:
for y in y_range:
for z in z_range:
plt.scatter(x, y, marker='o', color='blue')
# 绘制当前点
plt.scatter(current_point[0], current_point[1], marker='o', color='red')
# 更新当前点为下一个相邻点
next_x = np.random.choice([-1, 0, 1])
next_y = np.random.choice([-1, 0, 1])
next_z = np.random.choice([-0.1, 0, 0.1])
current_point = (current_point[0] + next_x, current_point[1] + next_y, current_point[2] + next_z)
# 创建动画
fig = plt.figure()
animation = FuncAnimation(fig, update, frames=100, interval=200)
plt.show()
```
运行以上代码,就可以看到一个动画绘制的过程,从网格的一个随机点开始依次访问相邻点,并在网格上移动。
### 回答3:
可以使用Python的matplotlib库来绘制这段动画描述过程。具体步骤如下:
1. 导入需要的库
```
import matplotlib.pyplot as plt
import numpy as np
import matplotlib.animation as animation
```
2. 定义坐标轴范围
```
x_range = [0, 10, 1]
y_range = [0, 10, 1]
z_range = [1.5, 1.6]
```
3. 创建坐标轴网格数据
```
x = np.arange(x_range[0], x_range[1] + x_range[2], x_range[2])
y = np.arange(y_range[0], y_range[1] + y_range[2], y_range[2])
z = np.arange(z_range[0], z_range[1] + z_range[2], z_range[2])
X, Y, Z = np.meshgrid(x, y, z)
```
4. 创建一个空的figure和3D坐标轴
```
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
```
5. 定义更新函数,用于每个时间步骤更新图形
```
def update(num):
# 清除旧的数据
ax.cla()
# 绘制新的数据点
ax.scatter(X.flatten(), Y.flatten(), Z.flatten(), c='blue', s=10)
ax.scatter(X.flatten()[num], Y.flatten()[num], Z.flatten()[num], c='red', s=20)
# 设置坐标轴范围和标签
ax.set_xlim(x_range[0], x_range[1])
ax.set_ylim(y_range[0], y_range[1])
ax.set_zlim(z_range[0], z_range[1])
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
# 设置标题
ax.set_title('Traversal Animation')
# 创建动画
ani = animation.FuncAnimation(fig, update, frames=X.shape[0] * X.shape[1] * X.shape[2], interval=200)
# 显示动画
plt.show()
```
以上代码中,update函数用于每个时间步骤更新图形,通过ax.scatter绘制网格中的数据点。ani = animation.FuncAnimation用于创建动画。运行代码后,即可看到描述过程的动画效果。
相关推荐
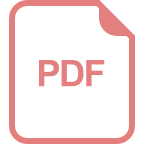
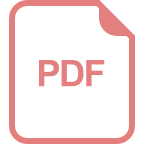
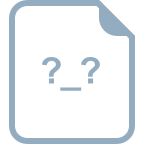











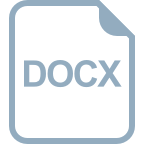
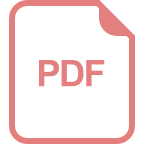
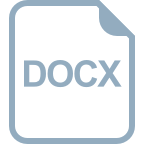
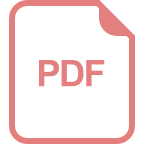