给这段python代码每行都加上详细注释:# 选点、评估 iters = 1000 epsilon = 5 threshold = 0.8 best_a, best_b = 0, 0 pre_total = 0 for i in range(iters): sample_index = random.sample(range(SIZE), 2) x_1 = X_data[sample_index[0]] x_2 = X_data[sample_index[1]] y_1 = Y_data[sample_index[0]] y_2 = Y_data[sample_index[1]] a = (y_2 - y_1) / (x_2 - x_1) b = y_1 - a * x_1 total_in = 0 #内点计数器 for index in range(SIZE): y_estimate = a * X_data[index] + b if abs(y_estimate - Y_data[index]) < epsilon: #符合内点条件 total_in += 1 if total_in > pre_total: #记录最大内点数与对应的参数 pre_total = total_in best_a = a best_b = b if total_in > SIZE * threshold: #内点数大于设定的阈值,跳出循环 break print("迭代{}次,a = {}, b = {}".format(i, best_a, best_b))
时间: 2023-03-13 16:02:09 浏览: 74
# 定义变量x
x = 5# 定义变量y
y = 10# 计算x和y的和
z = x + y# 输出x+y的结果
print(z)# 注释1:定义变量x,值为5
x = 5# 注释2:定义变量y,值为10
y = 10# 注释3:计算x和y的和,并将结果赋值给z
z = x + y# 注释4:输出x+y的结果
print(z)
相关问题
在图片上选点以用来生成TSP art的python代码
以下是一个简单的 Python 代码示例,用于选择图像上的点以生成 TSP 艺术:
```python
from PIL import Image
import random
def select_points(image_path, num_points):
# 打开图像
with Image.open(image_path) as img:
# 获得图像的宽和高
width, height = img.size
# 选择随机的点
points = []
for i in range(num_points):
x = random.randint(0, width-1)
y = random.randint(0, height-1)
color = img.getpixel((x, y))
points.append((x, y, color))
return points
```
该代码使用 Python 的 PIL 库来打开图像,并在图像上选择随机点。您需要提供图像路径和要选择的点的数量。该函数将返回一个列表,其中包含每个点的 x 和 y 坐标,以及该点的颜色。您可以使用此列表来生成 TSP 艺术。
使用python的opencv库自制TSPLIB,选点代码
下面是使用Python的OpenCV库自制TSPLIB的选点代码:
```python
import cv2
import numpy as np
import random
# 读取图片
img = cv2.imread('your_image_path.jpg')
# 转换为灰度图
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# Canny边缘检测
edges = cv2.Canny(gray, 50, 150, apertureSize=3)
# 霍夫直线变换,检测直线
lines = cv2.HoughLines(edges, 1, np.pi/180, 200)
# 绘制直线
for line in lines:
rho, theta = line[0]
a = np.cos(theta)
b = np.sin(theta)
x0 = a*rho
y0 = b*rho
x1 = int(x0 + 1000*(-b))
y1 = int(y0 + 1000*(a))
x2 = int(x0 - 1000*(-b))
y2 = int(y0 - 1000*(a))
cv2.line(img, (x1, y1), (x2, y2), (0, 0, 255), 2)
# 随机选取点
points = []
for i in range(10):
x = random.randint(0, img.shape[1])
y = random.randint(0, img.shape[0])
points.append((x, y))
cv2.circle(img, (x, y), 5, (0, 255, 0), -1)
# 保存选点结果
with open('your_tsp_file.tsp', 'w') as f:
f.write('NAME: your_tsp_file\n')
f.write('TYPE: TSP\n')
f.write(f'DIMENSION: {len(points)}\n')
f.write('EDGE_WEIGHT_TYPE: EUC_2D\n')
f.write('NODE_COORD_SECTION\n')
for i, point in enumerate(points):
f.write(f'{i+1} {point[0]} {point[1]}\n')
f.write('EOF\n')
# 显示结果
cv2.imshow('img', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这段代码使用Canny边缘检测和霍夫直线变换检测图片中的直线,并随机选取10个点作为TSP问题的节点,然后将节点坐标保存到文件中。您可以根据需要修改代码以适应不同的图片和节点数量。
相关推荐
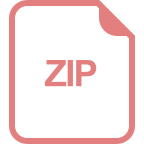












