2、建立一个新的工程,从英语:文本中构建单词表和出现次数的字典。 3、输出频率最高的10个单词,去除冠词、代词和介词等无意义的单词。 4、可以定义多个函数分别实现独立的功能。在主模块中串联各个函数,实现总体上的功能。
时间: 2024-09-12 21:07:45 浏览: 93
首先,要建立一个新的工程并从给定的文本中构建单词表和出现次数的字典,可以遵循以下步骤:
1. 创建一个新工程,并设置一个文本文件读取模块。这个模块负责读取输入的英语文本文件,并将其内容加载到内存中。
2. 接着,创建一个单词清洗和统计模块。这个模块将文本内容转换为单词列表,同时去除标点符号、数字和其他非单词字符。同时,它还需要移除冠词、代词、介词等常见的无意义单词(stop words)。
3. 然后,创建一个字典构建模块,用于统计剩余单词出现的次数,并将这些单词和对应的计数存储在一个字典中。
4. 最后,创建一个排序和输出模块,用于对字典中的单词按出现频率进行排序,并输出频率最高的10个单词。
以下是一个简化的代码示例,用于说明如何实现上述功能:
```python
import re
from collections import Counter
def read_text(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
return file.read()
def clean_and_split(text):
# 定义英文中常见的无意义单词列表
stop_words = set(['the', 'a', 'an', 'and', 'or', 'but', 'if', 'for', 'nor', 'so', 'yet', 'at', 'around', 'by', 'after', 'along', 'for', 'from', 'of', 'on', 'to', 'with', 'without'])
# 将文本转换为小写并分割成单词列表
words = re.findall(r'\b\w+\b', text.lower())
# 移除停用词
words = [word for word in words if word not in stop_words]
return words
def count_words(words):
return Counter(words)
def get_top_n_words(word_count, n=10):
return word_count.most_common(n)
# 主模块
def main():
file_path = 'your_text_file.txt' # 指定文本文件路径
text = read_text(file_path)
words = clean_and_split(text)
word_count = count_words(words)
top_words = get_top_n_words(word_count)
print(top_words)
# 运行主模块
if __name__ == "__main__":
main()
```
阅读全文
相关推荐
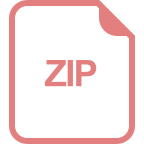
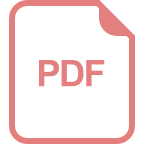
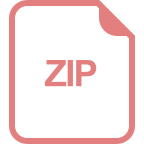






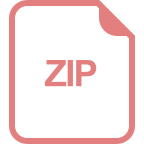
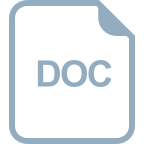
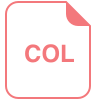
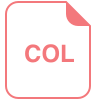
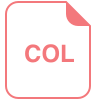
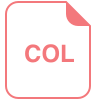
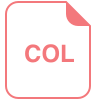
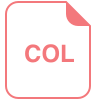
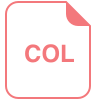
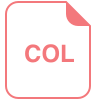