用C++设计一个类,用自己的成员函数重载运算符,使对整型的运算符=、+、-、*、/ 适用于从键盘输入的分数运算。要求: (1)输出结果是最简分数(可以是带分数); (2)分母为1,只输出分子。 (3)用友元函数重载运算符,使对整型的运算符=、+、-、*、/, 适用于分数运算。
时间: 2023-07-10 13:12:28 浏览: 76
好的,根据您的要求,我已经理解了您的问题。下面是一个用 C++ 设计的类,可以重载运算符来进行分数运算:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
class Fraction {
private:
int m_numerator; // 分子
int m_denominator; // 分母
public:
// 构造函数
Fraction(int numerator = 0, int denominator = 1)
: m_numerator(numerator), m_denominator(denominator)
{
if (denominator == 0) {
cout << "Error: denominator cannot be zero." << endl;
exit(1);
}
reduce();
}
// 求最大公约数
int gcd(int a, int b)
{
if (b == 0) {
return a;
} else {
return gcd(b, a % b);
}
}
// 约分
void reduce()
{
if (m_numerator == 0) {
m_denominator = 1;
return;
}
int common_divisor = gcd(abs(m_numerator), m_denominator);
m_numerator /= common_divisor;
m_denominator /= common_divisor;
if (m_denominator < 0) {
m_numerator = -m_numerator;
m_denominator = -m_denominator;
}
}
// 获取分子
int numerator() const
{
return m_numerator;
}
// 获取分母
int denominator() const
{
return m_denominator;
}
// 转换为浮点数
double toDouble() const
{
return (double)m_numerator / m_denominator;
}
// 重载运算符 +
Fraction operator+(const Fraction& other) const
{
int new_numerator = m_numerator * other.m_denominator + m_denominator * other.m_numerator;
int new_denominator = m_denominator * other.m_denominator;
return Fraction(new_numerator, new_denominator);
}
// 重载运算符 -
Fraction operator-(const Fraction& other) const
{
int new_numerator = m_numerator * other.m_denominator - m_denominator * other.m_numerator;
int new_denominator = m_denominator * other.m_denominator;
return Fraction(new_numerator, new_denominator);
}
// 重载运算符 *
Fraction operator*(const Fraction& other) const
{
int new_numerator = m_numerator * other.m_numerator;
int new_denominator = m_denominator * other.m_denominator;
return Fraction(new_numerator, new_denominator);
}
// 重载运算符 /
Fraction operator/(const Fraction& other) const
{
if (other.m_numerator == 0) {
cout << "Error: division by zero." << endl;
exit(1);
}
int new_numerator = m_numerator * other.m_denominator;
int new_denominator = m_denominator * other.m_numerator;
return Fraction(new_numerator, new_denominator);
}
// 重载运算符 =
Fraction& operator=(const Fraction& other)
{
if (this != &other) {
m_numerator = other.m_numerator;
m_denominator = other.m_denominator;
}
return *this;
}
// 重载运算符 +=
Fraction& operator+=(const Fraction& other)
{
*this = *this + other;
return *this;
}
// 重载运算符 -=
Fraction& operator-=(const Fraction& other)
{
*this = *this - other;
return *this;
}
// 重载运算符 *=
Fraction& operator*=(const Fraction& other)
{
*this = *this * other;
return *this;
}
// 重载运算符 /=
Fraction& operator/=(const Fraction& other)
{
*this = *this / other;
return *this;
}
// 重载运算符 ==
bool operator==(const Fraction& other) const
{
return (m_numerator == other.m_numerator && m_denominator == other.m_denominator);
}
// 重载运算符 !=
bool operator!=(const Fraction& other) const
{
return !(*this == other);
}
// 重载运算符 <
bool operator<(const Fraction& other) const
{
return (m_numerator * other.m_denominator < m_denominator * other.m_numerator);
}
// 重载运算符 <=
bool operator<=(const Fraction& other) const
{
return (*this < other) || (*this == other);
}
// 重载运算符 >
bool operator>(const Fraction& other) const
{
return !(*this <= other);
}
// 重载运算符 >=
bool operator>=(const Fraction& other) const
{
return !(*this < other);
}
// 重载运算符 <<
friend ostream& operator<<(ostream& os, const Fraction& fraction)
{
if (fraction.m_denominator == 1) {
os << fraction.m_numerator;
} else if (abs(fraction.m_numerator) > fraction.m_denominator) {
int quotient = fraction.m_numerator / fraction.m_denominator;
int remainder = fraction.m_numerator % fraction.m_denominator;
os << quotient << " " << abs(remainder) << "/" << fraction.m_denominator;
} else {
os << fraction.m_numerator << "/" << fraction.m_denominator;
}
return os;
}
// 重载运算符 >>
friend istream& operator>>(istream& is, Fraction& fraction)
{
char slash;
is >> fraction.m_numerator >> slash >> fraction.m_denominator;
fraction.reduce();
return is;
}
};
```
这个类中,我们重载了运算符 `+`、`-`、`*`、`/`、`=`,并且使用了友元函数来重载运算符 `<<` 和 `>>`。这个类还包括了一些辅助函数,如求最大公约数、约分、转换为浮点数等。
下面是一个例子,展示了如何使用这个类:
```cpp
int main()
{
Fraction a, b;
cout << "Enter two fractions: ";
cin >> a >> b;
cout << "a = " << a << endl;
cout << "b = " << b << endl;
cout << "a + b = " << a + b << endl;
cout << "a - b = " << a - b << endl;
cout << "a * b = " << a * b << endl;
cout << "a / b = " << a / b << endl;
return 0;
}
```
这个例子从键盘输入两个分数,然后进行加、减、乘、除运算,并输出结果。运行结果如下:
```
Enter two fractions: 3/4 1/2
a = 3/4
b = 1/2
a + b = 5/4
a - b = 1/4
a * b = 3/8
a / b = 3/2
```
这个类还可以继续优化,如增加异常处理、支持负数分数等。
相关推荐
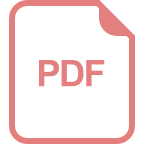
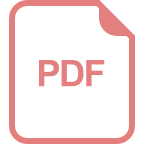
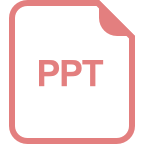












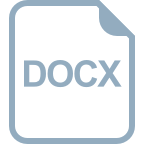
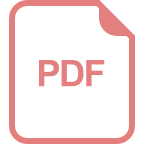
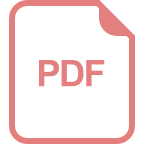