c++,设计和实现整型集合类(Set),使它成为一个类模板,并在主函数中实例化2种不同用法,整型集合类的成员函数要求如下: 添加构造函数完成初始化(可用一个集合或数组初始化另一个集合) 输出所有元素 添加析构函数释放内存 重载+,-和*运算符实现集合的并、差、交; 重载赋值运算符=,用一个集合给另一个集合赋值
时间: 2023-07-16 09:11:26 浏览: 142
以下是一个简单的整型集合类 Set 的实现示例,包括构造函数、析构函数、重载运算符等:
```cpp
#include <iostream>
#include <algorithm>
#include <iterator>
#include <vector>
template <typename T>
class Set {
public:
// 构造函数
Set() : data_{}, size_{0} {}
Set(std::vector<T> const& data) : size_{data.size()} {
std::copy(data.begin(), data.end(), std::back_inserter(data_));
}
// 拷贝构造函数
Set(Set const& other) : data_{other.data_}, size_{other.size_} {}
// 移动构造函数
Set(Set&& other) noexcept : data_{std::move(other.data_)}, size_{other.size_} {}
// 析构函数
~Set() { data_.clear(); }
// 重载运算符
Set& operator=(Set const& other) {
if (this == &other) return *this;
data_ = other.data_;
size_ = other.size_;
return *this;
}
Set& operator+=(Set const& other) {
std::vector<T> result(size_ + other.size_);
std::merge(data_.begin(), data_.end(), other.data_.begin(), other.data_.end(), result.begin());
data_ = result;
size_ = result.size();
return *this;
}
Set& operator-=(Set const& other) {
std::vector<T> result(size_);
auto it = std::set_difference(data_.begin(), data_.end(), other.data_.begin(), other.data_.end(), result.begin());
result.resize(it - result.begin());
data_ = result;
size_ = result.size();
return *this;
}
Set& operator*=(Set const& other) {
std::vector<T> result(size_);
auto it = std::set_intersection(data_.begin(), data_.end(), other.data_.begin(), other.data_.end(), result.begin());
result.resize(it - result.begin());
data_ = result;
size_ = result.size();
return *this;
}
// 输出所有元素
void print() const {
std::cout << "{ ";
std::copy(data_.begin(), data_.end(), std::ostream_iterator<T>{std::cout, " "});
std::cout << "}" << std::endl;
}
private:
std::vector<T> data_;
size_t size_;
};
// 重载运算符
template <typename T>
Set<T> operator+(Set<T> const& a, Set<T> const& b) {
Set<T> result(a);
result += b;
return result;
}
template <typename T>
Set<T> operator-(Set<T> const& a, Set<T> const& b) {
Set<T> result(a);
result -= b;
return result;
}
template <typename T>
Set<T> operator*(Set<T> const& a, Set<T> const& b) {
Set<T> result(a);
result *= b;
return result;
}
int main() {
Set<int> a{ {1, 2, 3} };
Set<int> b{ {3, 4, 5} };
Set<int> c{ b };
std::cout << "a: "; a.print();
std::cout << "b: "; b.print();
std::cout << "c: "; c.print();
c = a + b;
std::cout << "a + b: "; c.print();
c = a - b;
std::cout << "a - b: "; c.print();
c = a * b;
std::cout << "a * b: "; c.print();
return 0;
}
```
在主函数中,我们实例化了两个不同的整型集合类 Set<int>,并且对它们进行了加、减、乘运算,并输出了结果。
阅读全文
相关推荐
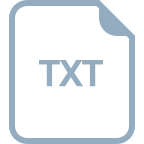
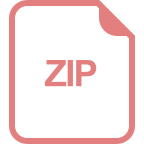
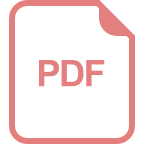

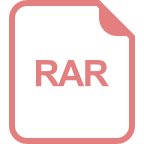
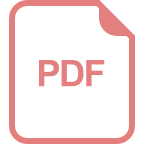
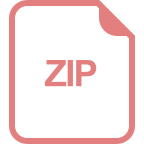
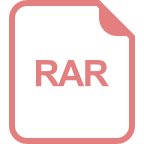
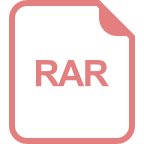
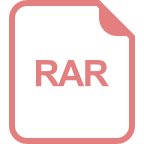
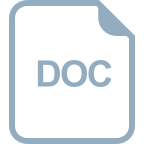
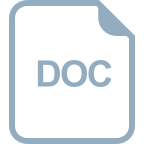
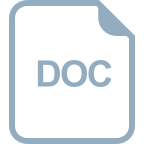
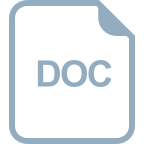
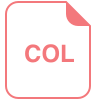
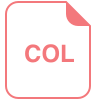
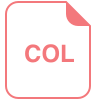
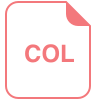