c++定义一个学生类,并在主函数声明学生类的一个对象
时间: 2023-05-18 17:05:34 浏览: 71
以下是一个简单的学生类的定义,包括姓名、年龄和成绩三个属性:
```c++
#include <iostream>
#include <string>
using namespace std;
class Student {
public:
string name;
int age;
double score;
};
int main() {
Student stu; // 声明一个学生对象
stu.name = "Tom";
stu.age = 18;
stu.score = 90.5;
cout << "Name: " << stu.name << endl;
cout << "Age: " << stu.age << endl;
cout << "Score: " << stu.score << endl;
return 0;
}
```
在主函数中,我们声明了一个名为`stu`的学生对象,并对其属性进行了赋值。最后输出了学生的姓名、年龄和成绩。
相关问题
运用友元关系定义一个学生的类和一个成绩的类,其中使得成绩类对象可以访问学生对象的私有数据,并编程实现(数据自定义)。
好的,这是一个关于 C++ 编程的问题。以下是示例代码:
class Student;
class Score {
public:
Score(): score_(0), student_(nullptr) {}
Score(int score): score_(score), student_(nullptr) {}
void SetScore(int score) {
score_ = score;
}
void SetStudent(Student* student) {
student_ = student;
}
void PrintScore() {
std::cout << "Score: " << score_ << std::endl;
std::cout << "Student name: " << student_->name_ << std::endl;
std::cout << "Student age: " << student_->age_ << std::endl;
}
private:
int score_;
Student* student_;
};
class Student {
public:
Student(): name_(""), age_(0) {}
Student(std::string name, int age): name_(name), age_(age) {}
friend class Score;
private:
std::string name_;
int age_;
};
int main() {
Student student1("Alice", 17);
Score score1(80);
score1.SetStudent(&student1);
score1.PrintScore();
return 0;
}
在上面的代码中,我们定义了一个学生类 Student 和一个成绩类 Score。在成绩类中,我们使用了友元关系,为了让成绩对象可以访问学生对象的私有数据。具体来说,我们将 Student 类声明为 Score 的友元类,这意味着 Score 类可以访问 Student 类中的私有成员。在 Score 类中,我们保存了一个指向学生对象的指针,这样我们可以访问学生对象的私有数据。在主函数中,我们创建了一个学生对象和一个成绩对象,并将学生对象的指针保存在成绩对象中。然后,我们调用 PrintScore() 函数来打印成绩和与之关联的学生的姓名和年龄。
希望这个示例代码可以帮助你理解如何使用友元关系来访问私有数据。
c++声明学生类Student,包含学号(id)、姓名(name)、成绩(score)等数据成员,成员函数有;构造函数输入学生信息、设置学生信息、输出学生信息函数等等。在主函数中定义3个对象,1个对象通过构造函数赋值,1个通过键盘输入、1个通过参数赋值,输出3个学生对象的所有数据成员;修改某个对象的成绩,输出该对象的成绩。
好的,下面是 C++ 的代码实现:
```cpp
#include <iostream>
#include <string>
using namespace std;
class Student {
private:
int id;
string name;
float score;
public:
// 构造函数输入学生信息
Student(int id = 0, string name = "", float score = 0.0)
: id(id), name(name), score(score) {}
// 设置学生信息
void setStudent(int id, string name, float score) {
this->id = id;
this->name = name;
this->score = score;
}
// 输出学生信息函数
void showStudent() {
cout << "学号:" << id << endl;
cout << "姓名:" << name << endl;
cout << "成绩:" << score << endl;
}
// 获取学生成绩
float getScore() {
return this->score;
}
// 修改学生成绩
void setScore(float score) {
this->score = score;
}
};
int main() {
// 通过构造函数赋值
Student s1(20190001, "张三", 90.0);
s1.showStudent();
cout << endl;
// 通过键盘输入
int id;
string name;
float score;
cout << "请输入学生信息(学号、姓名、成绩):" << endl;
cin >> id >> name >> score;
Student s2(id, name, score);
s2.showStudent();
cout << endl;
// 通过参数赋值
Student s3;
s3.setStudent(20190003, "李四", 80.0);
s3.showStudent();
cout << endl;
// 修改某个对象的成绩,输出该对象的成绩
float newScore;
cout << "请输入要修改成绩的学生的新成绩:" << endl;
cin >> newScore;
s1.setScore(newScore);
cout << "修改后的成绩为:" << s1.getScore() << endl;
return 0;
}
```
注意:这里假设学号为整数,姓名为字符串,成绩为浮点数。
相关推荐
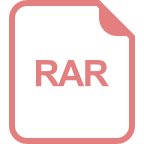
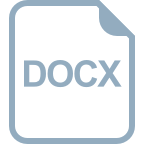
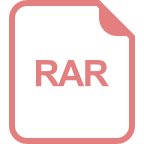












