使用c++编写学生类,分别为年龄、性别、各科成绩添加对应的Set函数,在函数内检测数据的合法性后再设置对应的成员变量值,如果有非法数据,则抛出异常,在主函数中产生学生对象并根据输入对其各属性赋值,捕获异常并提示对应的错误问题。
时间: 2024-03-19 12:45:13 浏览: 41
好的,我可以为您提供C++代码实现。
```c++
#include <iostream>
#include <string>
using namespace std;
class Student {
private:
int age;
string gender;
int mathScore, englishScore, chineseScore;
public:
void setAge(int age) {
if(age < 0 || age > 120) {
throw "Invalid age!";
}
this->age = age;
}
void setGender(string gender) {
if(gender != "male" && gender != "female") {
throw "Invalid gender!";
}
this->gender = gender;
}
void setMathScore(int score) {
if(score < 0 || score > 100) {
throw "Invalid math score!";
}
mathScore = score;
}
void setEnglishScore(int score) {
if(score < 0 || score > 100) {
throw "Invalid english score!";
}
englishScore = score;
}
void setChineseScore(int score) {
if(score < 0 || score > 100) {
throw "Invalid chinese score!";
}
chineseScore = score;
}
};
int main() {
Student student;
int age, mathScore, englishScore, chineseScore;
string gender;
try {
cout << "Please input the age: ";
cin >> age;
student.setAge(age);
cout << "Please input the gender: ";
cin >> gender;
student.setGender(gender);
cout << "Please input the math score: ";
cin >> mathScore;
student.setMathScore(mathScore);
cout << "Please input the english score: ";
cin >> englishScore;
student.setEnglishScore(englishScore);
cout << "Please input the chinese score: ";
cin >> chineseScore;
student.setChineseScore(chineseScore);
} catch(const char* e) {
cout << "Error: " << e << endl;
return 1;
}
cout << "Age: " << student.getAge() << endl;
cout << "Gender: " << student.getGender() << endl;
cout << "Math Score: " << student.getMathScore() << endl;
cout << "English Score: " << student.getEnglishScore() << endl;
cout << "Chinese Score: " << student.getChineseScore() << endl;
return 0;
}
```
在上述代码中,我们定义了一个 `Student` 类,其中包含 `age`、`gender`、`mathScore`、`englishScore` 和 `chineseScore` 这五个私有成员变量,并且为它们分别添加了对应的 `set` 函数。在 `set` 函数中,我们先检查传入的数据是否合法,如果合法则设置相应的成员变量值,否则抛出异常。
在 `main` 函数中,我们首先声明一个 `Student` 类型的对象 `student`,然后通过 `cin` 获取用户输入的数据,并且分别调用 `student` 对象的 `set` 函数进行赋值。如果在赋值过程中出现了异常,我们就通过 `catch` 捕获并输出错误信息。最后,我们输出 `student` 对象的各属性值。
阅读全文
相关推荐





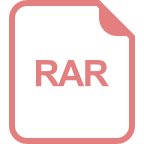



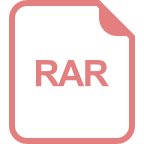








