用c++编写学生类,分别为年龄、性别、各科成绩添加对应的Set函数,在函数内检测数据的合法性后再设置对应的成员变量值,如果有非法数据,则抛出异常,在主函数中产生学生对象并根据输入对其各属性赋值,捕获异常并提示对应的错误问题。
时间: 2024-03-19 08:44:23 浏览: 49
好的,这是一个关于C++的编程问题。下面是一个示例代码,可以实现你的要求:
```cpp
#include <iostream>
#include <exception>
using namespace std;
class Student {
private:
int age;
char gender;
double mathScore;
double englishScore;
double chineseScore;
public:
void setAge(int a) {
if (a < 0 || a > 100) {
throw invalid_argument("Invalid age!");
}
age = a;
}
void setGender(char g) {
if (g != 'M' && g != 'F') {
throw invalid_argument("Invalid gender!");
}
gender = g;
}
void setMathScore(double s) {
if (s < 0 || s > 100) {
throw invalid_argument("Invalid math score!");
}
mathScore = s;
}
void setEnglishScore(double s) {
if (s < 0 || s > 100) {
throw invalid_argument("Invalid English score!");
}
englishScore = s;
}
void setChineseScore(double s) {
if (s < 0 || s > 100) {
throw invalid_argument("Invalid Chinese score!");
}
chineseScore = s;
}
};
int main() {
Student s;
int age;
char gender;
double mathScore, englishScore, chineseScore;
cout << "Enter age: ";
cin >> age;
try {
s.setAge(age);
}
catch (invalid_argument& e) {
cout << "Error: " << e.what() << endl;
return 1;
}
cout << "Enter gender (M/F): ";
cin >> gender;
try {
s.setGender(gender);
}
catch (invalid_argument& e) {
cout << "Error: " << e.what() << endl;
return 1;
}
cout << "Enter math score: ";
cin >> mathScore;
try {
s.setMathScore(mathScore);
}
catch (invalid_argument& e) {
cout << "Error: " << e.what() << endl;
return 1;
}
cout << "Enter English score: ";
cin >> englishScore;
try {
s.setEnglishScore(englishScore);
}
catch (invalid_argument& e) {
cout << "Error: " << e.what() << endl;
return 1;
}
cout << "Enter Chinese score: ";
cin >> chineseScore;
try {
s.setChineseScore(chineseScore);
}
catch (invalid_argument& e) {
cout << "Error: " << e.what() << endl;
return 1;
}
return 0;
}
```
在这个示例代码中,我们定义了一个名为`Student`的类,其中包含了年龄、性别和三个科目成绩的成员变量,以及对应的`set`函数。在每个`set`函数中,我们首先检查传入的参数是否合法,如果不合法则抛出一个`invalid_argument`类型的异常,并附带一个错误信息。在`main`函数中,我们依次输入每个属性的值,并尝试调用对应的`set`函数。如果函数抛出了异常,则我们捕获这个异常并输出对应的错误信息。如果没有异常,则说明赋值成功。
阅读全文
相关推荐




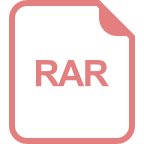



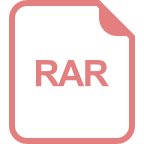








