如何通过C++ 实现逆向tf.nn.softmax的功能?要求:通过softmax的输出值,反推出softmax的输入值。
时间: 2023-11-29 18:16:26 浏览: 157
可以通过以下步骤来实现逆向tf.nn.softmax的功能:
1. 定义一个数组来存储softmax的输出值。
2. 对softmax的输出值进行归一化处理,使得所有值的和为1。
3. 对每个输出值进行反向操作,得到softmax的输入值。
以下是一个C++示例代码:
```c++
#include <iostream>
#include <vector>
#include <cmath>
using namespace std;
vector<float> inv_softmax(vector<float> output) {
int n = output.size();
float sum = 0.0;
vector<float> input(n, 0.0);
// 归一化处理
for (int i = 0; i < n; i++) {
sum += exp(output[i]);
}
for (int i = 0; i < n; i++) {
float exp_output = exp(output[i]);
input[i] = exp_output / sum;
// 反向操作
input[i] = log(input[i] / (1 - input[i]));
}
return input;
}
int main() {
vector<float> output = {0.1, 0.2, 0.3, 0.4};
vector<float> input = inv_softmax(output);
for (int i = 0; i < input.size(); i++) {
cout << input[i] << " ";
}
cout << endl;
return 0;
}
```
在该示例中,我们定义了一个`inv_softmax`函数,它接受一个包含softmax输出值的向量,并返回一个包含softmax输入值的向量。在函数中,我们首先对输出值进行归一化处理,然后对每个输出值进行反向操作,最后将结果存储在返回的输入向量中。在主函数中,我们对示例输出进行了测试。
阅读全文
相关推荐
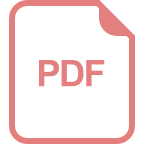
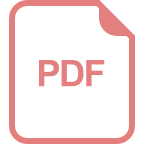
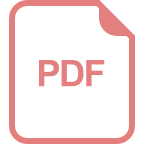




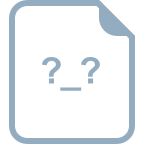
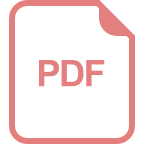
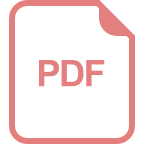
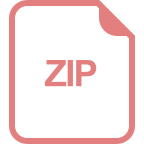
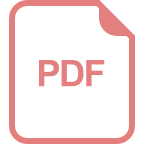
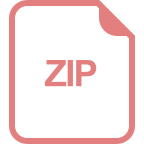
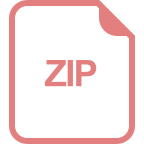
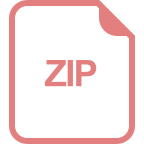
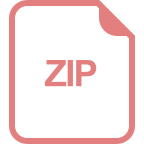
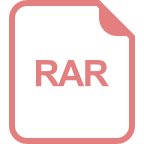
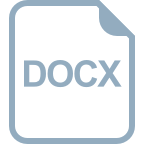