如何通过C++ 实现逆向tf.nn.softmax的功能?通过输入softmax的输出值,反推出输入到softmax的值。
时间: 2023-11-29 15:16:23 浏览: 78
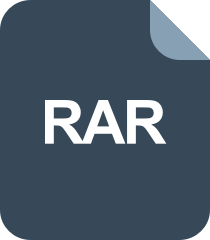
逆向c++
在C++中实现逆向tf.nn.softmax的功能需要使用数值计算库,如Eigen或者NumPy等。以下是一个简单的实现过程:
1. 首先,将softmax的输出值作为输入值,存储在一个向量中。
2. 对于输入向量中的每个值,计算其对应的概率值。
3. 然后,对每个概率值求导数,得到一个对应的Jacobian矩阵。
4. 最后,将Jacobian矩阵与softmax输出向量的转置相乘,得到输入向量的梯度。
以下是示例代码:
```c++
#include <iostream>
#include <Eigen/Dense>
using namespace Eigen;
MatrixXd inv_softmax(VectorXd softmax_output) {
int n = softmax_output.size();
MatrixXd jacobian(n, n);
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
if (i == j) {
jacobian(i, j) = softmax_output(i) * (1 - softmax_output(i));
} else {
jacobian(i, j) = -softmax_output(i) * softmax_output(j);
}
}
}
return jacobian * softmax_output.transpose();
}
int main() {
VectorXd softmax_output(3);
softmax_output << 0.3, 0.5, 0.2;
MatrixXd inv_softmax_output = inv_softmax(softmax_output);
std::cout << inv_softmax_output << std::endl;
return 0;
}
```
该示例代码中,我们使用Eigen库来进行数值计算,其中inv_softmax函数接受softmax输出向量作为输入,并返回输入向量的梯度。在主函数中,我们将一个示例softmax输出向量传递给inv_softmax函数,并输出计算得到的输入向量的梯度矩阵。
阅读全文
相关推荐




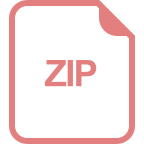
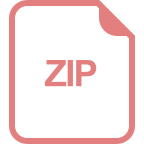
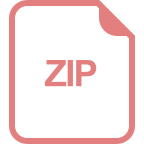
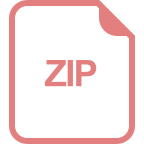
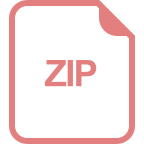
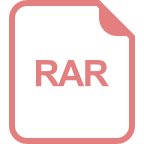
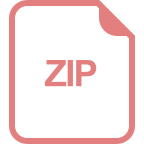
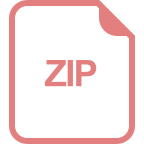
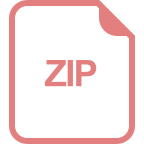