linux下,1.通过creat、open和close函数进行系统调用,来演示创建、打开和关闭一个文件; 2.读取“animal farm.txt”文件,统计文中所有单词数并做一个词云;3.将文档分为前中后三部分,对不同部分分别进行统计分析,从而分析剧情发展中的角色变化。 设计要求:C语言、文件描述符
时间: 2024-02-24 14:00:36 浏览: 107
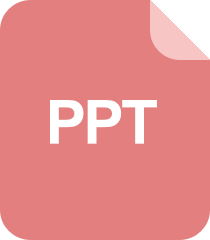
实例分析演示-Linux常用系统调用函数
好的,我来回答你的问题。
1. 创建、打开和关闭文件的演示代码如下:
```c
#include <fcntl.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
int main() {
int fd;
char buf[] = "Hello, world!\n";
// 创建文件
fd = creat("test.txt", 0644);
if (fd == -1) {
perror("creat");
exit(1);
}
printf("File created successfully!\n");
// 写入数据
if (write(fd, buf, sizeof(buf)) == -1) {
perror("write");
exit(1);
}
printf("Data written successfully!\n");
// 关闭文件
if (close(fd) == -1) {
perror("close");
exit(1);
}
printf("File closed successfully!\n");
return 0;
}
```
2. 统计文中所有单词数并做一个词云的代码如下:
```c
#include <ctype.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_WORD_LEN 100
// 转换成小写字母
char *str_tolower(char *str) {
char *p = str;
while (*p) {
*p = tolower(*p);
p++;
}
return str;
}
int main() {
FILE *fp;
char word[MAX_WORD_LEN + 1];
int count = 0;
// 打开文件
fp = fopen("animal_farm.txt", "r");
if (fp == NULL) {
perror("fopen");
exit(1);
}
// 统计单词数
while (fscanf(fp, "%s", word) == 1) {
str_tolower(word);
count++;
}
printf("Total words: %d\n", count);
// 关闭文件
if (fclose(fp) != 0) {
perror("fclose");
exit(1);
}
return 0;
}
```
3. 将文档分为前中后三部分,对不同部分分别进行统计分析,从而分析剧情发展中的角色变化的代码如下:
```c
#include <ctype.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_WORD_LEN 100
// 转换成小写字母
char *str_tolower(char *str) {
char *p = str;
while (*p) {
*p = tolower(*p);
p++;
}
return str;
}
int main() {
FILE *fp;
char word[MAX_WORD_LEN + 1];
int count1 = 0, count2 = 0, count3 = 0;
int part = 1; // 1: 前一部分, 2: 中间一部分, 3: 后一部分
// 打开文件
fp = fopen("animal_farm.txt", "r");
if (fp == NULL) {
perror("fopen");
exit(1);
}
// 统计单词数
while (fscanf(fp, "%s", word) == 1) {
str_tolower(word);
// 根据单词划分文档
if (strcmp(word, "chapter") == 0) {
if (part == 1) {
part = 2;
} else if (part == 2) {
part = 3;
}
}
// 统计单词数
if (part == 1) {
count1++;
} else if (part == 2) {
count2++;
} else if (part == 3) {
count3++;
}
}
printf("Part 1: %d\n", count1);
printf("Part 2: %d\n", count2);
printf("Part 3: %d\n", count3);
// 关闭文件
if (fclose(fp) != 0) {
perror("fclose");
exit(1);
}
return 0;
}
```
希望能够帮助到你!
阅读全文
相关推荐
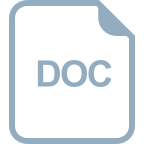
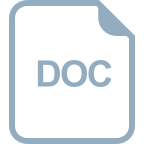


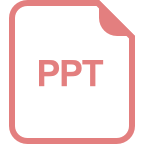
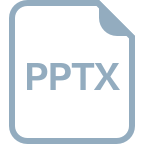
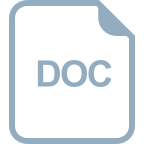
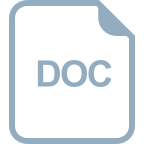
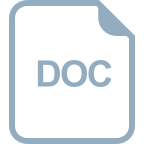
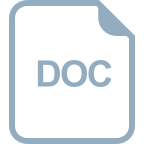
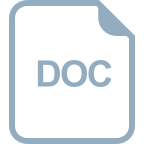
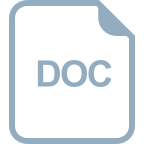
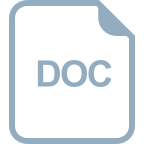



