linux环境通过creat、open和close函数进行系统调用,来演示创建、打开和关闭一个文件; (2) 读取“animal farm”txt文件,统计文中所有单词数和英文介词“in”、“on”、“at”的个数。
时间: 2023-09-16 17:12:46 浏览: 71
1. 创建、打开和关闭文件的演示代码如下:
```c
#include <stdio.h>
#include <fcntl.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <unistd.h>
int main() {
// 使用creat函数创建文件
int fd1 = creat("test1.txt", 0666);
if (fd1 < 0) {
perror("creat");
return -1;
}
printf("Created file test1.txt with file descriptor %d\n", fd1);
// 使用open函数打开文件
int fd2 = open("test2.txt", O_RDWR | O_CREAT, 0666);
if (fd2 < 0) {
perror("open");
return -1;
}
printf("Opened file test2.txt with file descriptor %d\n", fd2);
// 使用close函数关闭文件
close(fd1);
close(fd2);
printf("Closed files\n");
return 0;
}
```
2. 统计单词数和英文介词个数的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
int main() {
FILE *fp;
char filename[] = "animal farm.txt";
char word[100];
int word_count = 0, in_count = 0, on_count = 0, at_count = 0;
fp = fopen(filename, "r");
if (fp == NULL) {
perror("fopen");
exit(EXIT_FAILURE);
}
while (fscanf(fp, "%s", word) != EOF) {
word_count++;
if (strcmp(word, "in") == 0) {
in_count++;
} else if (strcmp(word, "on") == 0) {
on_count++;
} else if (strcmp(word, "at") == 0) {
at_count++;
}
}
printf("Total words: %d\n", word_count);
printf("in count: %d\n", in_count);
printf("on count: %d\n", on_count);
printf("at count: %d\n", at_count);
fclose(fp);
return 0;
}
```
需要注意的是,上述代码中使用了`strcmp`函数来比较字符串是否相等,而在比较前需要将字符串转换为小写字母,否则可能会出现不匹配的情况。另外,由于单词可能包含标点符号等其他字符,因此需要使用`ctype.h`库中的函数来进行判断和处理。
相关推荐
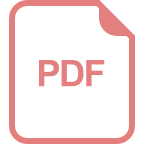
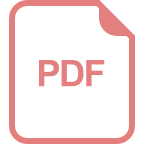
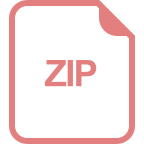














