使用Linux文件系统调用从a.txt中读100个字节复制到文件b.txt中
时间: 2024-06-13 20:05:26 浏览: 21
可以使用Linux文件系统调用中的open、read、write和close函数来实现从a.txt中读100个字节复制到文件b.txt中的操作。具体步骤如下:
1. 使用open函数打开a.txt文件,并以只读方式打开。
2. 使用open函数打开b.txt文件,并以写入方式打开。
3. 使用read函数从a.txt文件中读取100个字节的数据。
4. 使用write函数将读取的数据写入到b.txt文件中。
5. 重复步骤3和4,直到a.txt文件中的数据全部读取完毕。
6. 使用close函数关闭a.txt和b.txt文件。
下面是示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#define BUF_SIZE 100
int main()
{
int fd1, fd2, n;
char buf[BUF_SIZE];
// 打开a.txt文件
fd1 = open("a.txt", O_RDONLY);
if (fd1 == -1) {
perror("open a.txt");
exit(EXIT_FAILURE);
}
// 打开b.txt文件
fd2 = open("b.txt", O_WRONLY | O_CREAT | O_TRUNC, 0644);
if (fd2 == -1) {
perror("open b.txt");
exit(EXIT_FAILURE);
}
// 从a.txt文件中读取数据并写入到b.txt文件中
while ((n = read(fd1, buf, BUF_SIZE)) > 0) {
if (write(fd2, buf, n) != n) {
perror("write b.txt");
exit(EXIT_FAILURE);
}
}
// 关闭文件
if (close(fd1) == -1) {
perror("close a.txt");
exit(EXIT_FAILURE);
}
if (close(fd2) == -1) {
perror("close b.txt");
exit(EXIT_FAILURE);
}
return 0;
}
```
相关推荐
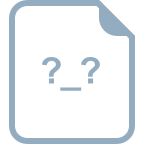
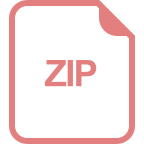
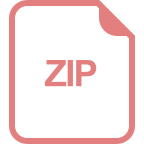














