avio_alloc_context 用法
时间: 2023-08-01 16:15:34 浏览: 122
avio_alloc_context 是 FFmpeg 中用于创建 AVIOContext 的函数。AVIOContext 是 FFmpeg 中的 IO 抽象层,用于封装各种输入输出操作,例如从文件读取数据、向网络发送数据等。avio_alloc_context 函数用于分配并初始化 AVIOContext 结构体,以便进行输入输出操作。
函数原型如下:
```
AVIOContext *avio_alloc_context(
unsigned char *buffer,
int buffer_size,
int write_flag,
void *opaque,
int (*read_packet)(void *opaque, uint8_t *buf, int buf_size),
int (*write_packet)(void *opaque, uint8_t *buf, int buf_size),
int64_t (*seek)(void *opaque, int64_t offset, int whence)
);
```
参数说明如下:
- `buffer`:指向用于缓存数据的内存地址。可以为 NULL,表示不需要缓存数据。
- `buffer_size`:缓存区的大小。如果 `buffer` 为 NULL,则该参数无效。
- `write_flag`:标志位,表示 AVIOContext 是否支持写操作。如果为 1,表示支持写操作;如果为 0,表示只支持读操作。
- `opaque`:指向用户自定义的数据结构的指针,用于在读写操作中传递额外的数据。
- `read_packet`:读数据的回调函数指针。函数原型为 `int (*read_packet)(void *opaque, uint8_t *buf, int buf_size)`,返回值为读取的字节数,如果读取失败则返回负数。
- `write_packet`:写数据的回调函数指针。函数原型为 `int (*write_packet)(void *opaque, uint8_t *buf, int buf_size)`,返回值为写入的字节数,如果写入失败则返回负数。
- `seek`:定位操作的回调函数指针。函数原型为 `int64_t (*seek)(void *opaque, int64_t offset, int whence)`,返回值为定位后的文件位置,如果定位失败则返回负数。
下面是一个简单的示例,演示如何使用 avio_alloc_context 函数创建 AVIOContext:
```c
#include <libavformat/avformat.h>
int main(int argc, char *argv[]) {
// 打开一个文件
FILE *file = fopen("test.mp4", "rb");
if (!file) {
printf("Failed to open file.\n");
return -1;
}
// 创建 AVIOContext
uint8_t *buffer = (uint8_t *)av_malloc(4096);
AVIOContext *avio_ctx = avio_alloc_context(buffer, 4096, 0, file, NULL, NULL, NULL);
if (!avio_ctx) {
printf("Failed to allocate AVIOContext.\n");
return -1;
}
// 打开输入格式上下文
AVFormatContext *fmt_ctx = avformat_alloc_context();
fmt_ctx->pb = avio_ctx;
int ret = avformat_open_input(&fmt_ctx, NULL, NULL, NULL);
if (ret < 0) {
printf("Failed to open input.\n");
return -1;
}
// 释放资源
avformat_close_input(&fmt_ctx);
av_free(avio_ctx->buffer);
av_free(avio_ctx);
return 0;
}
```
在该示例中,首先打开了一个文件。然后,创建 AVIOContext 的缓存区,并将文件指针作为 opaque 参数传递给 avio_alloc_context 函数。最后,将 AVIOContext 指定为输入格式上下文的 pb 字段,调用 avformat_open_input 函数打开输入。
需要注意的是,在使用完 AVIOContext 后,需要释放它所占用的内存空间,以避免内存泄漏。可以使用 av_free 函数释放缓存区,使用 avio_context_free 函数释放 AVIOContext 结构体。
阅读全文
相关推荐
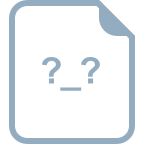
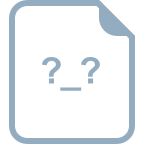


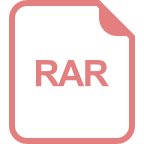
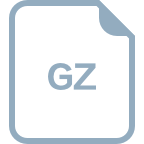
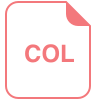







