<input type="hidden" id="customerTcId" value="${customerTcRelation.id}"/>如何避免${customerTcRelation.id}为NULL
时间: 2024-10-10 14:08:42 浏览: 14
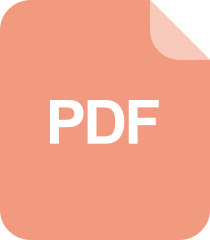
jQuery插件ajaxfileupload.js实现上传文件
在Java编程中,如果你正在处理数据库查询或操作,并且担心某个字段(如`customerTcId`)可能会因为某些原因(比如数据未填充、用户输入错误或查询失败)而为`NULL`,你可以采取以下策略来避免这种情况:
1. **预检查**:在使用`customerTcId`之前,先检查它是否已经赋值。例如,在获取数据后立即检查其值是否为`null`:
```java
CustomerTcRelation customerTcRelation = ... // 获取数据
if (customerTcRelation != null && customerTcRelation.getId() != null) {
int id = customerTcRelation.getId();
// 现在可以安全地使用id
}
```
2. **使用Optional**:Java 8引入了`Optional`类,可以帮助管理可能为`null`的返回值。将`customerTcId`包装成`Optional`,然后使用`orElse()`或`get()`提供默认值:
```java
Optional<CustomerTcRelation> optional = Optional.ofNullable(customerTcRelation);
int id = optional.map(CustomerTcRelation::getId).orElseGet(() -> defaultId);
```
3. **数据库查询优化**:在数据库查询时设置`NOT NULL`约束或者添加`COALESCE`或`IFNULL`函数(如果是在SQL查询),确保该字段总是有值。
4. **异常处理**:如果确实可能发生`NULL`值的情况,可以捕获并处理异常,而不是让程序崩溃:
```java
try {
int id = customerTcRelation.getId();
} catch (NullPointerException e) {
log.error("customerTcId is null", e);
// 处理异常情况,如返回默认值或者抛出一个新的异常
}
```
在实际应用中,选择哪种策略取决于具体的业务需求和上下文。
阅读全文
相关推荐
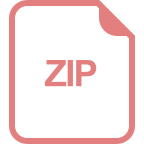
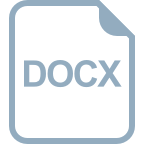










<% //连接数据库 String driver = "com.mysql.jdbc.Driver"; String url = "jdbc:mysql://localhost:3306/votedb?serverTimezone=UTC&characterEncoding=UTF-8"; String username = "root"; String password = "123456"; Connection conn = null; Statement stmt = null; ResultSet rs = null; try { Class.forName(driver); conn = DriverManager.getConnection(url, username, password); stmt = conn.createStatement(); rs = stmt.executeQuery("SELECT * FROM vote"); while(rs.next()){ String title = rs.getString("title"); %> <input type="radio" name="option" value="<%=title%>"><%=title%>
<% } } catch (Exception e) { e.printStackTrace(); } finally { //关闭连接 try { if (rs != null) { rs.close(); } if (stmt != null) { stmt.close(); } if (conn != null) { conn.close(); } } catch (SQLException e) { e.printStackTrace(); } } %> 提交投票选中后数据库数据变化
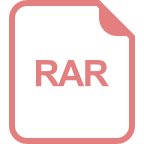




