在Python和OpenCV的支持下,如何从零开始构建一个简单有效的指纹识别系统?请提供具体的开发流程和实现代码。
时间: 2024-12-07 15:32:51 浏览: 17
构建一个指纹识别系统涉及多个步骤,包括图像采集、预处理、特征提取和匹配。以下是详细的实现流程和代码示例:
参考资源链接:[Python+OpenCV打造高效指纹识别系统教程](https://wenku.csdn.net/doc/5362i09wps?spm=1055.2569.3001.10343)
首先,确保安装了Python和OpenCV库。可以使用pip命令安装OpenCV:
pip install opencv-python
然后,编写代码来实现指纹识别系统的关键步骤:
1. 图像采集:
通常需要使用指纹扫描仪来获取图像数据。在这个例子中,我们假设已经有一张指纹图像。
2. 图像预处理:
对采集的图像进行预处理,包括灰度转换、滤波、二值化等,以减少噪声和增强特征。
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('fingerprint.jpg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 应用高斯模糊
blurred = cv2.GaussianBlur(gray, (5, 5), 0)
# 二值化处理
_, thresh = cv2.threshold(blurred, 120, 255, cv2.THRESH_BINARY_INV)
```
3. 特征提取:
使用OpenCV的模板匹配功能来识别指纹图像中的特征点。
```python
# 使用模板匹配算法寻找特征点
template = cv2.imread('fingerprint_template.jpg', 0)
w, h = template.shape[::-1]
res = cv2.matchTemplate(gray, template, cv2.TM_CCOEFF_NORMED)
min_val, max_val, min_loc, max_loc = cv2.minMaxLoc(res)
top_left = max_loc
bottom_right = (top_left[0] + w, top_left[1] + h)
cv2.rectangle(image, top_left, bottom_right, 255, 2)
```
4. 特征匹配和验证:
将提取的特征与数据库中的模板进行比对,验证身份。
```python
# 假设我们有一个函数来加载和比对指纹模板
def match_fingerprint(template_fingerprint, scanned_fingerprint):
# 比对指纹逻辑
pass
# 假设模板指纹已经加载到数据库
db_template = load_template_from_db('user_template.jpg')
matched = match_fingerprint(db_template, thresh)
if matched:
print(
参考资源链接:[Python+OpenCV打造高效指纹识别系统教程](https://wenku.csdn.net/doc/5362i09wps?spm=1055.2569.3001.10343)
阅读全文
相关推荐
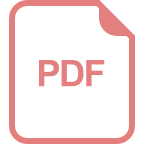
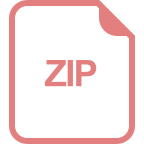
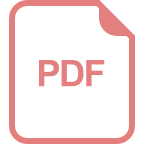
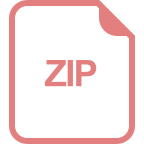








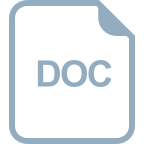
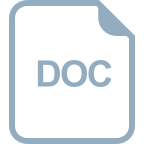
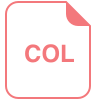
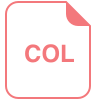
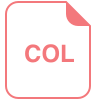

