用python写一个可运行的国际象棋
时间: 2023-05-18 19:04:40 浏览: 61
游戏程序,包括棋盘、棋子、移动规则和胜利条件的实现。以下是一个简单的示例代码:
```python
class ChessBoard:
def __init__(self):
self.board = [['R', 'N', 'B', 'Q', 'K', 'B', 'N', 'R'],
['P', 'P', 'P', 'P', 'P', 'P', 'P', 'P'],
[' ', '.', ' ', '.', ' ', '.', ' ', '.'],
['.', ' ', '.', ' ', '.', ' ', '.', ' '],
[' ', '.', ' ', '.', ' ', '.', ' ', '.'],
['.', ' ', '.', ' ', '.', ' ', '.', ' '],
['p', 'p', 'p', 'p', 'p', 'p', 'p', 'p'],
['r', 'n', 'b', 'q', 'k', 'b', 'n', 'r']]
def print_board(self):
for row in self.board:
print(' '.join(str(x) for x in row))
class ChessPiece:
def __init__(self, color):
self.color = color
class Pawn(ChessPiece):
def __init__(self, color):
super().__init__(color)
self.symbol = 'P'
class Knight(ChessPiece):
def __init__(self, color):
super().__init__(color)
self.symbol = 'N'
class Bishop(ChessPiece):
def __init__(self, color):
super().__init__(color)
self.symbol = 'B'
class Rook(ChessPiece):
def __init__(self, color):
super().__init__(color)
self.symbol = 'R'
class Queen(ChessPiece):
def __init__(self, color):
super().__init__(color)
self.symbol = 'Q'
class King(ChessPiece):
def __init__(self, color):
super().__init__(color)
self.symbol = 'K'
class ChessGame:
def __init__(self):
self.board = ChessBoard()
self.turn = 'white'
def play(self):
while True:
self.board.print_board()
move = input('Enter move: ')
if move == 'quit':
break
# TODO: implement move validation and execution
self.turn = 'black' if self.turn == 'white' else 'white'
if __name__ == '__main__':
game = ChessGame()
game.play()
```
这个程序实现了一个简单的国际象棋游戏,包括棋盘和棋子的表示,以及玩家输入和回合制的实现。但是它还没有实现移动规则和胜利条件,需要进一步完善。
相关推荐
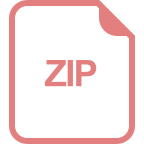
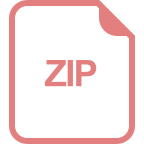
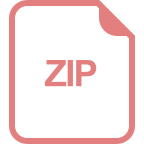









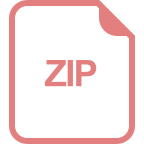
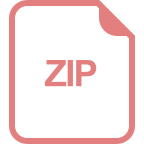
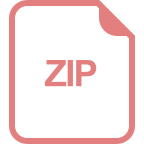
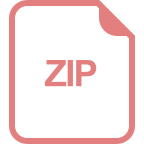
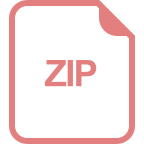
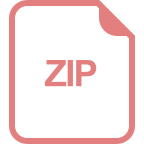