winform如何根据三维坐标绘制三维曲线
时间: 2023-09-05 09:06:48 浏览: 234
要在WinForm中绘制三维曲线,你可以使用OpenGL或DirectX等图形渲染库。这些库可以让你在三维空间中绘制对象和曲线。下面是一个使用OpenGL进行三维曲线绘制的示例代码:
1. 首先,你需要在WinForm中添加一个OpenGL控件。可以使用OpenTK库来实现:
```
using OpenTK.Graphics.OpenGL;
using OpenTK;
public partial class Form1 : Form
{
private GLControl glControl;
public Form1()
{
InitializeComponent();
glControl = new GLControl();
this.Controls.Add(glControl);
}
}
```
2. 然后,你需要在OpenGL控件的Paint事件中绘制三维曲线。使用glBegin(GL_LINE_STRIP)函数启动绘制线段的过程,使用glVertex3f(x, y, z)函数指定每个点的坐标,使用glEnd()函数结束绘制。
```
private void glControl_Paint(object sender, PaintEventArgs e)
{
GL.ClearColor(Color.Black);
GL.Clear(ClearBufferMask.ColorBufferBit | ClearBufferMask.DepthBufferBit);
GL.MatrixMode(MatrixMode.Projection);
GL.LoadIdentity();
GL.Viewport(0, 0, glControl.Width, glControl.Height);
GL.Perspective(45.0f, (float)glControl.Width / (float)glControl.Height, 0.1f, 100.0f);
GL.MatrixMode(MatrixMode.Modelview);
GL.LoadIdentity();
GL.Translate(0.0f, 0.0f, -6.0f);
GL.Begin(BeginMode.LineStrip);
for (float x = -1.0f; x <= 1.0f; x += 0.01f)
{
float y = (float)Math.Sin(x * 10.0f) * 0.5f;
float z = x;
GL.Vertex3(x, y, z);
}
GL.End();
glControl.SwapBuffers();
}
```
这个例子绘制了一条沿着x轴的三维正弦曲线。你可以修改顶点的坐标来绘制其他曲线。
阅读全文
相关推荐
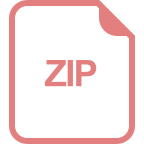
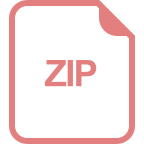
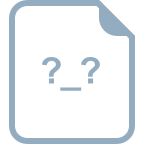


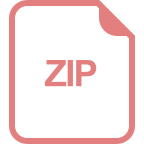
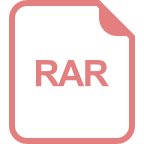
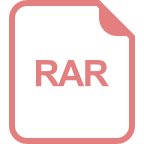
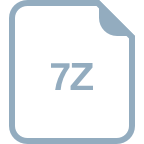
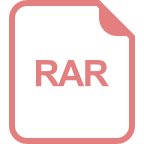
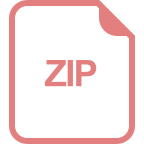
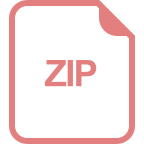
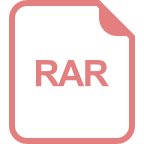
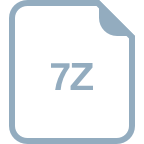
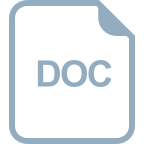
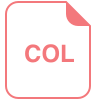

