java 写一个rsa密码算法
时间: 2023-11-18 17:18:52 浏览: 44
好的,以下是 Java 实现的 RSA 密码算法:
```java
import java.math.BigInteger;
import java.util.Random;
public class RSA {
private BigInteger privateKey;
private BigInteger publicKey;
private BigInteger modulus;
public void generateKeys(int bitLength) {
Random random = new Random();
BigInteger p = BigInteger.probablePrime(bitLength / 2, random);
BigInteger q = BigInteger.probablePrime(bitLength / 2, random);
BigInteger n = p.multiply(q);
BigInteger phi = p.subtract(BigInteger.ONE).multiply(q.subtract(BigInteger.ONE));
BigInteger e = BigInteger.valueOf(65537);
BigInteger d = e.modInverse(phi);
publicKey = e;
privateKey = d;
modulus = n;
}
public byte[] encrypt(byte[] message) {
BigInteger m = new BigInteger(1, message);
BigInteger c = m.modPow(publicKey, modulus);
return c.toByteArray();
}
public byte[] decrypt(byte[] message) {
BigInteger c = new BigInteger(1, message);
BigInteger m = c.modPow(privateKey, modulus);
return m.toByteArray();
}
public static void main(String[] args) {
RSA rsa = new RSA();
rsa.generateKeys(1024);
String message = "Hello, world!";
byte[] encrypted = rsa.encrypt(message.getBytes());
byte[] decrypted = rsa.decrypt(encrypted);
System.out.println("Original message: " + message);
System.out.println("Encrypted message: " + new String(encrypted));
System.out.println("Decrypted message: " + new String(decrypted));
}
}
```
在这个实现中,`generateKeys` 方法用于生成密钥对,`encrypt` 方法用于加密明文,`decrypt` 方法用于解密密文。在 `main` 方法中,我们生成密钥对并使用它们来加密和解密一条消息。
需要注意的是,这个实现中使用的是固定的公钥指数(65537)。在实际应用中,可以使用更多的方法来选择一个安全的公钥指数。另外,该实现也没有考虑填充(padding)和消息认证(message authentication)等问题。在实际应用中,需要使用更加完整的 RSA 实现来确保安全性。
相关推荐
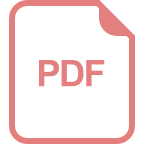
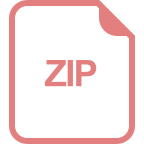
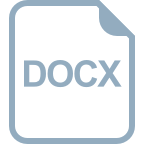














