Python中以地底点与待检测点连线方向作为起始搜索方向,以从地底点到待检测点的连线作为搜索路径,将该搜索路径上的所有地底点依次进行检测
时间: 2024-05-02 16:21:48 浏览: 12
。具体实现可以使用以下步骤:
1. 计算地底点与待检测点的连线方向,可以使用向量相减和计算夹角的方式得到。
2. 将该连线方向作为搜索方向,使用深度优先搜索或广度优先搜索算法进行搜索。
3. 在搜索过程中,记录搜索路径上的所有地底点。
4. 对每个搜索路径上的地底点进行检测,可以使用Ray Casting算法或其他方法。
5. 如果检测到待检测点,说明搜索路径上所有地底点都可见,否则需要继续搜索其他方向上的路径。
以下是一个简单的示例代码,实现了上述步骤:
```python
import numpy as np
def detect_underground_points(start_point, end_point, underground_points):
# 计算连线方向
direction = end_point - start_point
direction /= np.linalg.norm(direction)
# 搜索路径
path = [start_point]
# 搜索过程
while True:
# 沿着连线方向搜索
next_point = path[-1] + direction
path.append(next_point)
# 检测所有地底点
all_visible = True
for p in underground_points:
if is_point_visible(path[-2], path[-1], p):
continue
else:
all_visible = False
break
# 如果所有地底点都可见,则搜索结束
if all_visible:
break
# 返回搜索路径上的所有地底点
return path[1:]
def is_point_visible(start_point, end_point, underground_point):
# 判断地底点是否在起点和终点之间
if np.dot(underground_point - start_point, end_point - start_point) < 0:
return False
if np.dot(underground_point - end_point, start_point - end_point) < 0:
return False
# 判断地底点是否在起点和终点的连线上
if np.cross(end_point - start_point, underground_point - start_point) != 0:
return False
# 判断地底点和起点、终点的位置关系
if np.dot(end_point - start_point, underground_point - start_point) < 0:
return False
if np.dot(start_point - end_point, underground_point - end_point) < 0:
return False
# 地底点可见
return True
```
这个函数接受三个参数:起点、终点和地底点列表。它会返回搜索路径上的所有地底点。使用时,可以将这些点标记出来,以帮助用户理解地下结构。
相关推荐
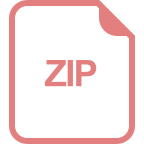
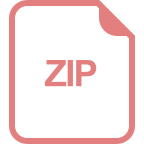
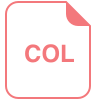
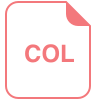
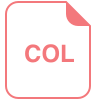
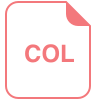
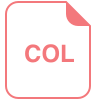









