编写setup.py文件,指定需要打包的Python文件和输出的DLL文件名
时间: 2024-05-05 18:19:42 浏览: 215
好的,以下是一个示例的setup.py文件,其中包含了需要打包的Python文件和输出的DLL文件名的设置:
```python
from distutils.core import setup, Extension
# 指定需要打包的Python文件
module1 = Extension('my_module', sources=['my_module.c'])
# 指定输出的DLL文件名
setup(name='my_module',
version='1.0',
description='My module',
ext_modules=[module1])
```
在这个示例中,我们使用了`Extension`类来指定需要打包的Python文件,其中`my_module`是输出的DLL文件名,`my_module.c`是需要打包的Python文件。我们将`Extension`对象传递给`ext_modules`参数,最终使用`setup()`函数来创建打包文件。
相关问题
我现在想用py2exe打包一个程序,要以管理员权限运行,无图标,版本为6.6.6.6,不显示窗口,文件名@NOTHING@.py
您可以按照以下步骤使用py2exe打包符合您要求的程序:
1. 安装py2exe
在命令行中运行以下命令安装py2exe:
```
pip install py2exe
```
2. 创建setup.py文件
在Python程序的根目录中创建一个名为`setup.py`的文件,并添加以下内容:
```python
from distutils.core import setup
import py2exe
import sys
sys.argv.append('py2exe')
setup(
options={
"py2exe":
{
"compressed": 1,
"optimize": 2,
"bundle_files": 1,
"dll_excludes": ["w9xpopen.exe"],
"uac_info": "requireAdministrator",
"version": "6.6.6.6",
"name": "@NOTHING@",
"description": "@NOTHING@",
"icon_resources": [(0, "")],
}
},
windows=[{'script': '@NOTHING@.py', "show_window": False}],
zipfile=None
)
```
将上面的`@NOTHING@`替换为您想要的程序名称。
在上面的代码中,我们将`options`参数设置为py2exe的选项,其中包括:
- `compressed`:是否压缩文件
- `optimize`:优化等级
- `bundle_files`:是否将所有文件打包到一个可执行文件中
- `dll_excludes`:需要排除的dll文件
- `uac_info`:设置需要管理员权限运行
- `version`:设置程序的版本号
- `name`:设置程序名称
- `description`:设置程序描述
- `icon_resources`:设置程序图标
3. 打包程序
在命令行中运行以下命令以将程序打包为可执行文件:
```
python setup.py py2exe
```
打包完成后,您可以在`dist`文件夹中找到可执行文件。
请注意,由于我们在代码中将窗口设置为不显示,因此您将无法在运行程序时看到任何输出。如果您想要查看程序的输出,请将代码中的`"show_window": False`改为`"show_window": True`。
把python的py打包成dll ,包括所有相关的库
好的,下面我将为你提供一个比较简单的实现方式,仅供参考。在这个例子中,我们将使用Python自带的ctypes模块来将Python代码打包成DLL文件。
假设我们要将以下Python代码打包成DLL文件:
```python
# test.py
import numpy as np
def add(a, b):
return np.add(a, b)
```
那么我们可以按照以下步骤进行操作:
1. 安装Python的开发包,以便在Windows系统下编译Python扩展模块。可以从Python官网下载对应版本的开发包。
2. 安装Microsoft Visual C++ Build Tools,以便在Windows系统下编译C/C++代码。
3. 在命令行中进入Python的安装目录下的Scripts文件夹,执行以下命令,安装setuptools和wheel:
```
pip install setuptools wheel
```
4. 在命令行中进入Python代码所在的目录,执行以下命令,打包Python代码为扩展模块:
```
python setup.py bdist_wheel
```
其中,setup.py文件的内容如下:
```python
from setuptools import setup, Extension
import numpy as np
ext_module = Extension(
'_test',
sources=['test.c', 'test_wrap.c'],
include_dirs=[np.get_include()],
libraries=['python36'],
library_dirs=[r'C:\Python36\libs'],
extra_compile_args=['/openmp']
)
setup(
name='test',
version='1.0',
ext_modules=[ext_module]
)
```
其中,'_test'表示生成的扩展模块名称,sources列表中包括了Python代码和用于将Python代码转换成C代码的包装器(test_wrap.c)。include_dirs需要包括NumPy库的头文件路径,libraries需要包括Python库文件名,library_dirs需要包括Python库文件路径。
5. 在命令行中进入dist文件夹,执行以下命令,将扩展模块打包成DLL文件:
```
python -m pip install wheel
python -m pip install twine
twine check dist/*
```
6. 将生成的DLL文件复制到其他程序中,并使用ctypes模块进行加载和调用。例如,在C++程序中,可以使用以下代码:
```c++
// main.cpp
#include <windows.h>
#include <iostream>
typedef int (*AddFunc)(int*, int*, int*, int);
int main()
{
// 加载DLL文件
HINSTANCE hDll = LoadLibrary("test.cp36-win_amd64.pyd");
if (!hDll) {
std::cout << "Load DLL failed!" << std::endl;
return 1;
}
// 获取函数指针
AddFunc addFunc = (AddFunc)GetProcAddress(hDll, "add");
if (!addFunc) {
std::cout << "Get function failed!" << std::endl;
return 1;
}
// 准备参数
int a[3] = {1, 2, 3};
int b[3] = {4, 5, 6};
int c[3];
// 调用函数
addFunc(a, b, c, 3);
for (int i = 0; i < 3; ++i) {
std::cout << c[i] << " ";
}
std::cout << std::endl;
// 卸载DLL文件
FreeLibrary(hDll);
return 0;
}
```
其中,test.cp36-win_amd64.pyd是生成的DLL文件名。需要注意的是,Python的扩展模块使用了GIL(Global Interpreter Lock)来控制线程安全,因此在多线程环境下可能会有性能瓶颈。如果需要高性能的调用,可以使用类似Cython的工具将Python代码编译成C/C++代码,再打包成DLL文件。
阅读全文
相关推荐
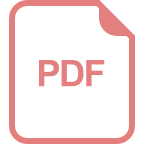
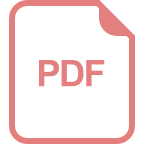
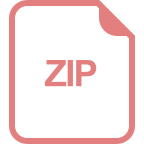
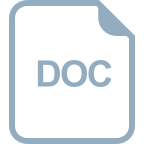
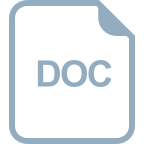
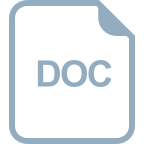
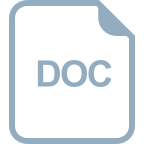
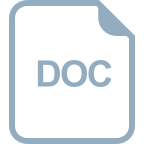
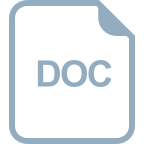
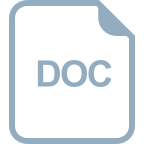
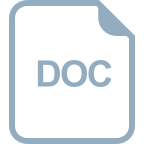
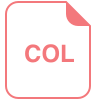



