python 根据key去value json
时间: 2023-11-27 14:03:27 浏览: 38
可以使用Python内置的`json`模块来解析JSON数据,并根据 key 值获取对应的 value。示例代码如下:
```python
import json
# JSON 数据
data = '{"name": "John", "age": 30, "city": "New York"}'
# 将JSON数据解析为Python对象
obj = json.loads(data)
# 根据 key 值获取对应的 value
print(obj["name"]) # 输出:John
print(obj["age"]) # 输出:30
print(obj["city"]) # 输出:New York
```
如果JSON数据很大,可以使用`json.load()`函数从文件中加载JSON数据。例如:
```python
import json
# 从文件中加载JSON数据
with open('data.json', 'r') as f:
data = json.load(f)
# 根据 key 值获取对应的 value
print(data["name"])
```
其中,`data.json`是包含JSON数据的文件名。
相关问题
python获取json key value戴安
可以使用Python内置的`json`模块来处理JSON数据。以下是一个简单的示例代码,可以获取JSON中的键和值:
```python
import json
# 一个JSON字符串
json_str = '{"name": "David", "age": 30, "city": "Beijing"}'
# 将JSON字符串解析为Python对象
json_obj = json.loads(json_str)
# 遍历Python对象,获取键和值
for key, value in json_obj.items():
print(key, value)
```
输出结果为:
```
name David
age 30
city Beijing
```
以上代码中,`json.loads()`函数将JSON字符串解析为Python对象,`json_obj.items()`方法获取键值对,再使用`for`循环遍历输出键和值。
python获取json的key和value
可以使用Python内置的json模块来获取JSON格式数据的key和value。可以通过以下方式实现:
```python
import json
# 将JSON数据转换为Python数据结构
data = json.loads(json_string)
# 遍历Python数据结构来获取所有的key和value
for key, value in data.items():
print("Key:", key)
print("Value:", value)
```
其中,`json_string`是包含JSON数据的字符串变量,`json.loads()`方法将JSON字符串转换为Python数据结构,`data.items()`可以迭代获取Python字典中的所有key和value。
相关推荐
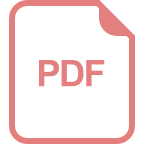
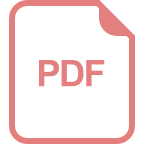
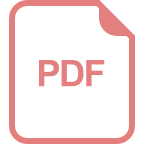
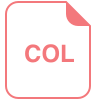
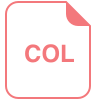
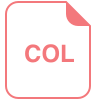
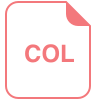
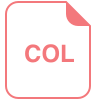







