使用python设计一款五子棋游戏,要求有GUI界面,给出代码实现
时间: 2024-03-31 12:38:15 浏览: 52
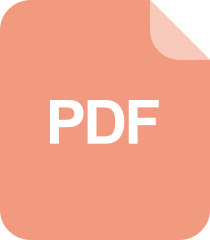
python五子棋游戏的设计与实现

好的,下面是五子棋游戏的代码实现:
```python
from tkinter import *
from tkinter.messagebox import *
import numpy as np
class Chessboard(Frame):
def __init__(self, master):
super().__init__(master)
self.chessboard = np.zeros((15, 15))
self.color = 1 # 黑棋先手
self.gameover = False
self.create_widgets()
def create_widgets(self):
self.canvas = Canvas(self, width=450, height=450, bg="white")
for i in range(15):
self.canvas.create_line(15, 15 + i * 30, 435, 15 + i * 30, width=2)
self.canvas.create_line(15 + i * 30, 15, 15 + i * 30, 435, width=2)
self.canvas.pack(side=LEFT)
self.button1 = Button(self, text="重新开始", command=self.restart)
self.button1.pack(side=TOP, pady=10)
self.button2 = Button(self, text="悔棋", command=self.undo)
self.button2.pack(side=TOP, pady=10)
self.button3 = Button(self, text="退出游戏", command=self.quit)
self.button3.pack(side=TOP, pady=10)
self.canvas.bind("<Button-1>", self.play)
def restart(self):
self.chessboard = np.zeros((15, 15))
self.color = 1
self.gameover = False
self.canvas.delete("all")
for i in range(15):
self.canvas.create_line(15, 15 + i * 30, 435, 15 + i * 30, width=2)
self.canvas.create_line(15 + i * 30, 15, 15 + i * 30, 435, width=2)
def undo(self):
if self.gameover:
showinfo("提示", "游戏已结束,无法悔棋!")
return
if len(self.history) <= 1:
showinfo("提示", "无棋可悔!")
return
self.chessboard[self.history[-1]] = 0
self.color = 3 - self.color
self.history.pop()
self.canvas.delete("last")
if self.color == 1:
showinfo("提示", "黑方悔棋!")
else:
showinfo("提示", "白方悔棋!")
def play(self, event):
if self.gameover:
showinfo("提示", "游戏已结束,无法落子!")
return
x, y = event.x, event.y
row, col = round((y-15)/30), round((x-15)/30)
if row < 0 or row > 14 or col < 0 or col > 14:
return
if self.chessboard[row][col] != 0:
return
self.canvas.create_oval(15+col*30-13, 15+row*30-13, 15+col*30+13, 15+row*30+13, fill="black" if self.color == 1 else "white")
self.chessboard[row][col] = self.color
self.history.append((row, col))
if self.check(row, col, self.color):
self.gameover = True
showinfo("提示", "黑棋胜!" if self.color == 1 else "白棋胜!")
return
self.color = 3 - self.color
if self.color == 1:
showinfo("提示", "轮到黑方落子!")
else:
showinfo("提示", "轮到白方落子!")
def check(self, row, col, color):
count = 1
for i in range(1, 5):
if row-i >= 0 and self.chessboard[row-i][col] == color:
count += 1
else:
break
for i in range(1, 5):
if row+i <= 14 and self.chessboard[row+i][col] == color:
count += 1
else:
break
if count >= 5:
return True
count = 1
for i in range(1, 5):
if col-i >= 0 and self.chessboard[row][col-i] == color:
count += 1
else:
break
for i in range(1, 5):
if col+i <= 14 and self.chessboard[row][col+i] == color:
count += 1
else:
break
if count >= 5:
return True
count = 1
for i in range(1, 5):
if row-i >= 0 and col-i >= 0 and self.chessboard[row-i][col-i] == color:
count += 1
else:
break
for i in range(1, 5):
if row+i <= 14 and col+i <= 14 and self.chessboard[row+i][col+i] == color:
count += 1
else:
break
if count >= 5:
return True
count = 1
for i in range(1, 5):
if row-i >= 0 and col+i <= 14 and self.chessboard[row-i][col+i] == color:
count += 1
else:
break
for i in range(1, 5):
if row+i <= 14 and col-i >= 0 and self.chessboard[row+i][col-i] == color:
count += 1
else:
break
if count >= 5:
return True
return False
class Game:
def __init__(self):
self.root = Tk()
self.root.title("五子棋")
self.chessboard = Chessboard(self.root)
self.chessboard.pack(side=LEFT, padx=10, pady=10)
self.chessboard.history = [(-1, -1)]
self.root.mainloop()
if __name__ == "__main__":
game = Game()
```
在代码中,我们使用了numpy库来表示棋盘状态,使用Tkinter库来实现GUI界面。在Chessboard类中,我们定义了棋盘的大小、棋盘状态、棋子颜色、游戏是否结束以及棋子落子等方法。在play方法中,我们判断棋子是否落在了空位上,并且判断落子后是否出现了五子连珠。在undo方法中,我们可以悔棋操作,通过一个叫做history的列表来记录每一步落子的位置。在restart方法中,我们可以重新开始游戏。最后,在Game类中,我们创建了一个窗口并将棋盘加入到其中。
阅读全文
相关推荐
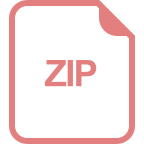



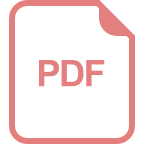
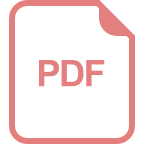
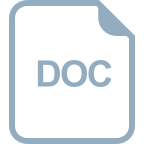
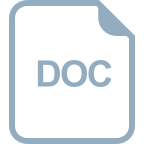
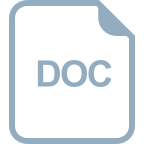
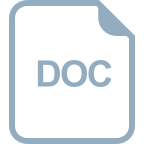
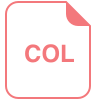





