python设计五子棋游戏gui
时间: 2023-09-15 13:15:28 浏览: 49
好的,下面是一个基于Python tkinter库设计的五子棋游戏GUI的示例代码:
```python
import tkinter as tk
from tkinter import messagebox
class Gobang():
def __init__(self, master):
self.master = master
self.master.title("五子棋")
self.master.geometry("600x600")
self.master.resizable(width=False, height=False)
self.current_player = "black"
self.game_over = False
self.chess_board = tk.Canvas(self.master, width=520, height=520, bg="pink")
self.chess_board.place(x=40, y=40)
for i in range(15):
self.chess_board.create_line(20 + i * 34, 20, 20 + i * 34, 500, width=2)
self.chess_board.create_line(20, 20 + i * 34, 500, 20 + i * 34, width=2)
self.chess_board.bind("<Button-1>", self.play)
self.reset_button = tk.Button(self.master, text="重置", command=self.reset)
self.reset_button.place(x=260, y=10)
def play(self, event):
if self.game_over:
return
x, y = event.x, event.y
if x < 20 or x > 500 or y < 20 or y > 500:
return
row, col = (y - 20) // 34, (x - 20) // 34
if self.board[row][col] != "":
return
self.board[row][col] = self.current_player
self.draw_piece(row, col, self.current_player)
if self.check_win(row, col):
messagebox.showinfo("游戏结束", f"{self.current_player} 赢了!")
self.game_over = True
return
if self.check_tie():
messagebox.showinfo("游戏结束", "平局!")
self.game_over = True
return
self.current_player = "white" if self.current_player == "black" else "black"
def draw_piece(self, row, col, player):
x, y = 20 + col * 34, 20 + row * 34
if player == "black":
self.chess_board.create_oval(x - 15, y - 15, x + 15, y + 15, fill="black")
else:
self.chess_board.create_oval(x - 15, y - 15, x + 15, y + 15, fill="white")
def check_win(self, row, col):
count = 1
for c in range(col - 1, -1, -1):
if self.board[row][c] == self.current_player:
count += 1
else:
break
for c in range(col + 1, 15):
if self.board[row][c] == self.current_player:
count += 1
else:
break
if count >= 5:
return True
count = 1
for r in range(row - 1, -1, -1):
if self.board[r][col] == self.current_player:
count += 1
else:
break
for r in range(row + 1, 15):
if self.board[r][col] == self.current_player:
count += 1
else:
break
if count >= 5:
return True
count = 1
for r, c in zip(range(row - 1, -1, -1), range(col - 1, -1, -1)):
if self.board[r][c] == self.current_player:
count += 1
else:
break
for r, c in zip(range(row + 1, 15), range(col + 1, 15)):
if self.board[r][c] == self.current_player:
count += 1
else:
break
if count >= 5:
return True
count = 1
for r, c in zip(range(row - 1, -1, -1), range(col + 1, 15)):
if self.board[r][c] == self.current_player:
count += 1
else:
break
for r, c in zip(range(row + 1, 15), range(col - 1, -1, -1)):
if self.board[r][c] == self.current_player:
count += 1
else:
break
if count >= 5:
return True
return False
def check_tie(self):
for row in range(15):
for col in range(15):
if self.board[row][col] == "":
return False
return True
def reset(self):
self.current_player = "black"
self.game_over = False
self.board = [["" for _ in range(15)] for _ in range(15)]
self.chess_board.delete("all")
for i in range(15):
self.chess_board.create_line(20 + i * 34, 20, 20 + i * 34, 500, width=2)
self.chess_board.create_line(20, 20 + i * 34, 500, 20 + i * 34, width=2)
if __name__ == "__main__":
root = tk.Tk()
game = Gobang(root)
root.mainloop()
```
这个五子棋游戏GUI有以下功能:
- 点击棋盘落子
- 判断胜负
- 判断平局
- 重置游戏
运行上面的代码,你会看到一个基于Python tkinter库的五子棋游戏GUI界面。
相关推荐
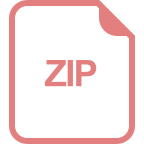
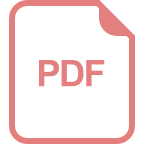
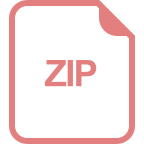














