C语言写出随机生成100以内的随机数并存入顺序表
时间: 2023-08-01 13:06:13 浏览: 130
好的,这是一个简单的C语言程序,可以生成100个0到99之间的随机数,并存储到顺序表中:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define MAXSIZE 100
typedef struct {
int data[MAXSIZE];
int length;
} SqList;
void InitList(SqList *L) {
L->length = 0;
}
void InsertList(SqList *L, int i, int e) {
if (i < 1 || i > L->length + 1 || L->length == MAXSIZE) {
return;
}
for (int j = L->length; j >= i; j--) {
L->data[j] = L->data[j - 1];
}
L->data[i - 1] = e;
L->length++;
}
int main() {
SqList L;
InitList(&L);
srand(time(NULL)); // 初始化随机数种子
for (int i = 0; i < MAXSIZE; i++) {
int num = rand() % 100; // 生成0到99之间的随机数
InsertList(&L, i + 1, num);
}
// 输出生成的随机数
for (int i = 0; i < L.length; i++) {
printf("%d ", L.data[i]);
}
printf("\n");
return 0;
}
```
程序中使用了顺序表来存储随机数,其中`InitList`函数用于初始化顺序表,`InsertList`函数用于在指定位置插入元素,然后在`main`函数中使用`rand`函数生成随机数,并调用`InsertList`函数将随机数存储到顺序表中,最后输出生成的随机数。
阅读全文
相关推荐






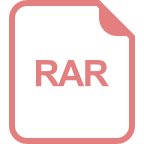



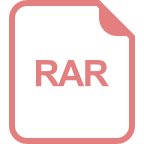

