springboot实现网页图片缓存到本地
时间: 2023-05-24 11:07:02 浏览: 320
下面是springboot实现网页图片缓存到本地的步骤:
1. 在pom.xml文件中添加以下依赖:
```
<!-- Spring Boot Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Spring Boot Thymeleaf -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!-- Apache Commons IO -->
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.6</version>
</dependency>
```
2. 创建一个名为FileUtil的工具类,用于保存图片到本地文件系统中:
```
import org.apache.commons.io.FileUtils;
import org.springframework.web.multipart.MultipartFile;
import java.io.File;
import java.io.IOException;
public class FileUtil {
public static void saveImage(MultipartFile image, String path, String filename) throws IOException {
File file = new File(path);
if (!file.exists()) {
file.mkdirs();
}
FileUtils.copyInputStreamToFile(image.getInputStream(), new File(path, filename));
}
}
```
3. 在Controller中添加以下方法,用于下载网页中的图片并保存到本地:
```
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.net.URL;
import java.util.List;
import java.util.UUID;
@Controller
public class HomeController {
@Value("${image.path}")
private String imagePath;
@GetMapping("/")
public String index() {
return "index";
}
@PostMapping("/")
public String uploadImages(@RequestParam("url") String url, Model model) throws IOException {
List<String> imageUrls = HTMLUtil.getImageUrls(url);
for (String imageUrl : imageUrls) {
URL imageLink = new URL(imageUrl);
String imageFileType = imageUrl.substring(imageUrl.lastIndexOf('.') + 1);
String imageName = UUID.randomUUID().toString() + "." + imageFileType;
FileUtil.saveImage(imageLink.openStream(), imagePath, imageName);
}
model.addAttribute("message", "Images saved successfully");
return "index";
}
}
```
4. 在application.properties文件中添加以下配置,用于指定保存图片的目录:
```
image.path=/tmp/images/
```
5. 创建一个名为HTMLUtil的工具类,用于从网页中提取图片的链接:
```
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
public class HTMLUtil {
public static List<String> getImageUrls(String url) throws IOException {
Document doc = Jsoup.connect(url).get();
List<String> imageUrls = new ArrayList<>();
doc.select("img").forEach(element -> {
String src = element.attr("src");
if (!src.startsWith("http")) {
src = "http://" + src;
}
imageUrls.add(src);
});
return imageUrls;
}
}
```
现在,当用户提交一个网址时,程序会提取出网页中的所有图片,将它们缓存到本地目录中。
相关推荐
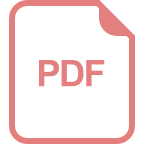
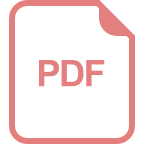
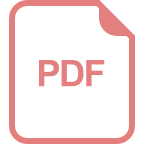













