springboot cache实现mybatis缓存注解
时间: 2023-06-12 15:02:47 浏览: 60
在Spring Boot中,我们可以使用`@Cacheable`注解来实现MyBatis缓存。以下是一个简单的示例:
1. 添加依赖
在`pom.xml`文件中添加以下依赖:
```xml
<dependency>
<groupId>org.mybatis.caches</groupId>
<artifactId>mybatis-ehcache</artifactId>
<version>1.2.1</version>
</dependency>
```
这里我们使用了Ehcache作为缓存提供者。
2. 配置缓存
在`application.properties`文件中添加以下配置:
```properties
mybatis.configuration.cache-enabled=true
mybatis.configuration.cache-impl=org.mybatis.caches.ehcache.EhcacheCache
```
这里我们启用了MyBatis的缓存,并将缓存实现设置为EhcacheCache。
3. 编写Mapper
```java
@Mapper
public interface UserMapper {
@Cacheable(value = "user", key = "'user_'+#id")
User getUserById(Long id);
}
```
在这个示例中,我们使用了`@Cacheable`注解来标记方法,让MyBatis自动缓存查询结果。`value`属性表示缓存的名称,`key`属性表示缓存的键。在这个例子中,我们使用了`id`作为键。
4. 测试
```java
@RunWith(SpringRunner.class)
@SpringBootTest
public class UserMapperTests {
@Autowired
private UserMapper userMapper;
@Test
public void testGetUserById() {
Long id = 1L;
User user = userMapper.getUserById(id);
System.out.println(user);
user = userMapper.getUserById(id);
System.out.println(user);
}
}
```
在这个测试中,我们首先查询了一个用户,然后再次查询同一个用户。由于缓存已经生效,第二次查询将直接从缓存中获取结果。
这就是使用Spring Boot Cache实现MyBatis缓存的简单示例。
相关推荐
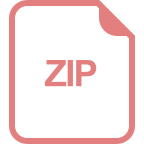
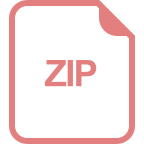
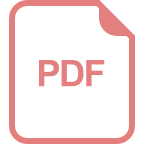














