请编写函数,从文件中读取成绩不及格的学生信息,将结果写入另一个文件。
时间: 2024-05-12 14:18:58 浏览: 85
这里提供Python代码实现:
```
def write_failing_students(input_file, output_file):
with open(input_file, 'r') as f_in, open(output_file, 'w') as f_out:
for line in f_in:
student_info = line.strip().split()
if int(student_info[1]) < 60: # 判断成绩是否不及格
f_out.write(line)
```
函数`write_failing_students`接受两个参数,分别为输入文件路径和输出文件路径。函数通过`with`语句打开输入文件和输出文件,遍历输入文件的每一行,将学生信息转化为列表形式,并对成绩进行判断。如果成绩不及格,就将这一行信息写入输出文件中。最后,函数自动关闭文件。
相关问题
请用C语言编写函数,从文件中读取成绩不及格的学生信息,将结果写入另一个文件。
以下是一个可能的实现:
```c
#include <stdio.h>
typedef struct {
char name[20];
int score;
} Student;
void write_failed_students(const char* input_filename, const char* output_filename) {
FILE* input_file = fopen(input_filename, "r");
if (input_file == NULL) {
printf("Failed to open input file %s\n", input_filename);
return;
}
FILE* output_file = fopen(output_filename, "w");
if (output_file == NULL) {
fclose(input_file);
printf("Failed to open output file %s\n", output_filename);
return;
}
Student student;
while (fscanf(input_file, "%s %d", student.name, &student.score) != EOF) {
if (student.score < 60) {
fprintf(output_file, "%s %d\n", student.name, student.score);
}
}
fclose(input_file);
fclose(output_file);
}
int main() {
write_failed_students("input.txt", "output.txt");
return 0;
}
```
这个程序定义了一个 `Student` 结构体表示学生,包含一个名字和一个分数。`write_failed_students` 函数接受两个文件名参数,打开输入文件并逐行读取学生信息,如果该学生的分数不及格(小于60),则将该学生的信息写入输出文件。最后,两个文件都会被关闭。在 `main` 函数中,我们调用 `write_failed_students` 来执行这个操作。
用c语言写:姓名 高等数学 英语 计算机 电学 张三 98 77 88 67 李四 88 90 99 56 王五 67 76 58 77 关六 66 77 77 80(2)建立一个数据文件st.txt,将以上学生成绩数据写入文件中; (3)从文件st.txt中读数据,计算每个学生的总成绩,并按总成绩排序,结果写入文件st_s.dat中; (4)查找并输出不及格学生的姓名、相应的课程名和成绩。
以下是用C语言实现题目要求的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_STUDENT_NUM 100
#define SUBJECT_NUM 4
typedef struct {
char name[20];
int scores[SUBJECT_NUM];
int total_score;
} Student;
void write_student_data_to_file(Student students[], int n, char* filename) {
FILE* fp = fopen(filename, "w");
if (fp == NULL) {
printf("Failed to open file %s for writing.\n", filename);
exit(1);
}
for (int i = 0; i < n; i++) {
fprintf(fp, "%s %d %d %d %d\n", students[i].name, students[i].scores[0], students[i].scores[1], students[i].scores[2], students[i].scores[3]);
}
fclose(fp);
printf("Successfully wrote student data to file %s.\n", filename);
}
void read_student_data_from_file(Student students[], int* n, char* filename) {
FILE* fp = fopen(filename, "r");
if (fp == NULL) {
printf("Failed to open file %s for reading.\n", filename);
exit(1);
}
*n = 0;
while (!feof(fp)) {
fscanf(fp, "%s %d %d %d %d", students[*n].name, &students[*n].scores[0], &students[*n].scores[1], &students[*n].scores[2], &students[*n].scores[3]);
int sum = 0;
for (int i = 0; i < SUBJECT_NUM; i++) {
sum += students[*n].scores[i];
}
students[*n].total_score = sum;
(*n)++;
}
(*n)--;
fclose(fp);
printf("Successfully read student data from file %s.\n", filename);
}
void sort_students_by_total_score(Student students[], int n) {
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if (students[i].total_score < students[j].total_score) {
Student temp = students[i];
students[i] = students[j];
students[j] = temp;
}
}
}
}
void write_sorted_student_data_to_file(Student students[], int n, char* filename) {
FILE* fp = fopen(filename, "w");
if (fp == NULL) {
printf("Failed to open file %s for writing.\n", filename);
exit(1);
}
for (int i = 0; i < n; i++) {
fprintf(fp, "%s %d %d %d %d %d\n", students[i].name, students[i].scores[0], students[i].scores[1], students[i].scores[2], students[i].scores[3], students[i].total_score);
}
fclose(fp);
printf("Successfully wrote sorted student data to file %s.\n", filename);
}
void find_failed_students(Student students[], int n) {
printf("Failed students:\n");
for (int i = 0; i < n; i++) {
for (int j = 0; j < SUBJECT_NUM; j++) {
if (students[i].scores[j] < 60) {
printf("%s %s %d\n", students[i].name, j == 0 ? "Math" : j == 1 ? "English" : j == 2 ? "Computer" : "Electric", students[i].scores[j]);
}
}
}
}
int main() {
Student students[MAX_STUDENT_NUM];
int n = 4;
strcpy(students[0].name, "张三");
students[0].scores[0] = 98;
students[0].scores[1] = 77;
students[0].scores[2] = 88;
students[0].scores[3] = 67;
strcpy(students[1].name, "李四");
students[1].scores[0] = 88;
students[1].scores[1] = 90;
students[1].scores[2] = 99;
students[1].scores[3] = 56;
strcpy(students[2].name, "王五");
students[2].scores[0] = 67;
students[2].scores[1] = 76;
students[2].scores[2] = 58;
students[2].scores[3] = 77;
strcpy(students[3].name, "关六");
students[3].scores[0] = 66;
students[3].scores[1] = 77;
students[3].scores[2] = 77;
students[3].scores[3] = 80;
write_student_data_to_file(students, n, "st.txt");
read_student_data_from_file(students, &n, "st.txt");
sort_students_by_total_score(students, n);
write_sorted_student_data_to_file(students, n, "st_s.dat");
find_failed_students(students, n);
return 0;
}
```
其中,`write_student_data_to_file()`函数将学生数据写入文件;`read_student_data_from_file()`函数从文件中读取学生数据,并计算每个学生的总成绩;`sort_students_by_total_score()`函数按照总成绩对学生进行排序;`write_sorted_student_data_to_file()`函数将排序后的学生数据写入文件;`find_failed_students()`函数查找并输出不及格学生的姓名、相应的课程名和成绩。主函数中,我们先手动初始化了学生数据,然后调用上述函数完成题目要求。
阅读全文
相关推荐
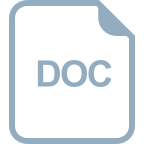
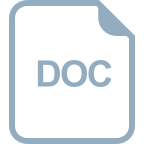
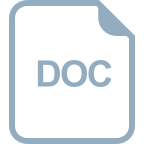
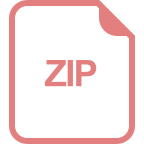












