如何在MFC中使用行程编码压缩图片,图片格式为bmp
时间: 2024-02-09 10:08:36 浏览: 66
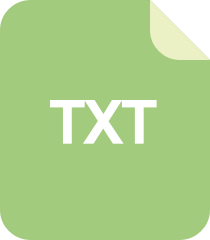
基于行程编码的图像压缩算法
在MFC中使用行程编码压缩BMP图片,可以使用 Windows 提供的 GDI+ 库,该库提供了对 BMP 图片的支持,并且可以使用 Run-Length Encoding(RLE)算法对 BMP 图片进行压缩。
下面是一个简单的示例代码,演示了如何使用 GDI+ 库实现 BMP 图片的 RLE 压缩和解压缩:
```cpp
#include <gdiplus.h>
#pragma comment(lib, "gdiplus.lib")
using namespace Gdiplus;
// 压缩 BMP 图片
bool CompressBmp(LPCTSTR lpszSrcFile, LPCTSTR lpszDstFile)
{
// 加载 BMP 图片
Bitmap bmp(lpszSrcFile);
if (bmp.GetLastStatus() != Ok)
{
return false;
}
// 获取 BMP 图片的位图信息头
BITMAPINFOHEADER bih;
bmp.GetHBITMAP(NULL, &bih);
// 创建压缩后的位图
Bitmap dstBmp(bih.biWidth, bih.biHeight, PixelFormat16bppRGB565);
// 获取压缩后的位图的位图信息头
BITMAPINFOHEADER dstBih;
dstBmp.GetHBITMAP(NULL, &dstBih);
// 创建压缩器
CLSID clsidEncoder;
GetEncoderClsid(L"image/bmp", &clsidEncoder);
EncoderParameters encoderParams;
encoderParams.Count = 1;
encoderParams.Parameter[0].Guid = EncoderCompression;
encoderParams.Parameter[0].Type = EncoderParameterValueTypeLong;
encoderParams.Parameter[0].NumberOfValues = 1;
encoderParams.Parameter[0].Value = &EncoderValueCompressionRle;
// 压缩 BMP 图片
Graphics g(&dstBmp);
g.DrawImage(&bmp, 0, 0, bih.biWidth, bih.biHeight);
dstBmp.Save(lpszDstFile, &clsidEncoder, &encoderParams);
return true;
}
// 解压缩 BMP 图片
bool DecompressBmp(LPCTSTR lpszSrcFile, LPCTSTR lpszDstFile)
{
// 加载压缩的 BMP 图片
Bitmap srcBmp(lpszSrcFile);
if (srcBmp.GetLastStatus() != Ok)
{
return false;
}
// 获取压缩的 BMP 图片的位图信息头
BITMAPINFOHEADER bih;
srcBmp.GetHBITMAP(NULL, &bih);
// 创建解压缩后的位图
Bitmap dstBmp(bih.biWidth, bih.biHeight, PixelFormat24bppRGB);
// 获取解压缩后的位图的位图信息头
BITMAPINFOHEADER dstBih;
dstBmp.GetHBITMAP(NULL, &dstBih);
// 创建解压缩器
CLSID clsidDecoder;
GetDecoderClsid(L"image/bmp", &clsidDecoder);
// 解压缩 BMP 图片
Graphics g(&dstBmp);
g.DrawImage(&srcBmp, 0, 0, bih.biWidth, bih.biHeight);
dstBmp.Save(lpszDstFile, &clsidDecoder, NULL);
return true;
}
```
上面的示例代码中,使用了 GDI+ 库提供的 `Bitmap` 类来加载、创建和保存 BMP 图片。在压缩 BMP 图片时,使用了 `EncoderParameters` 结构体来指定使用 RLE 算法进行压缩。
在解压缩 BMP 图片时,直接使用 `DrawImage` 方法将压缩的 BMP 图片绘制到解压缩后的位图上即可。
注意,使用 GDI+ 库需要在程序初始化时调用 `GdiplusStartup` 函数,在程序退出时调用 `GdiplusShutdown` 函数进行清理。
阅读全文
相关推荐
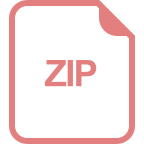
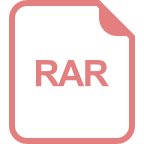
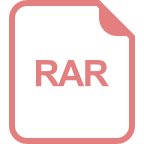
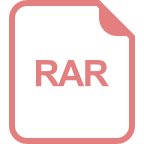
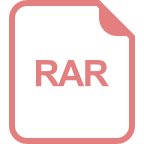
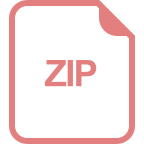
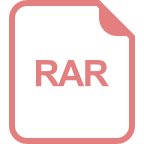
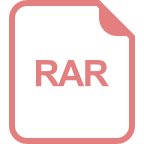
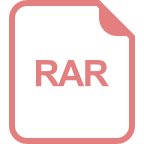
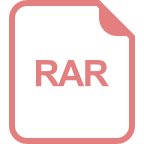
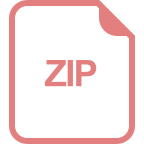
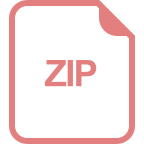
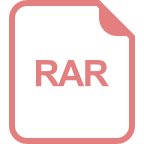
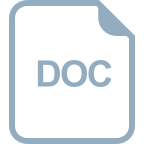