go语言实现解析字符串
时间: 2023-07-07 11:40:14 浏览: 159
在 Go 语言中,可以使用 strings 包提供的函数来解析字符串。以下是一些常用的函数:
1. strings.Split(s, sep):将字符串 s 按照分隔符 sep 进行分割,返回一个字符串数组。
2. strings.Trim(s, cutset):将字符串 s 去掉 cutset 中指定的字符。
3. strings.Replace(s, old, new, n):将字符串 s 中的 old 替换为 new,n 指定替换的次数,-1 表示全部替换。
4. strings.HasPrefix(s, prefix):判断字符串 s 是否以 prefix 开头。
5. strings.HasSuffix(s, suffix):判断字符串 s 是否以 suffix 结尾。
6. strings.Index(s, substr):查找字符串 s 中 substr 的位置,返回第一个匹配的位置,如果没有匹配则返回 -1。
7. strings.LastIndex(s, substr):查找字符串 s 中 substr 的位置,返回最后一个匹配的位置,如果没有匹配则返回 -1。
示例代码:
```go
package main
import (
"fmt"
"strings"
)
func main() {
// 分割字符串
str := "hello,world"
arr := strings.Split(str, ",")
fmt.Println(arr) // [hello world]
// 去掉指定字符
str2 := "hello,world\n"
str2 = strings.Trim(str2, "\n")
fmt.Println(str2) // hello,world
// 替换字符串
str3 := "hello,world"
str3 = strings.Replace(str3, "world", "golang", -1)
fmt.Println(str3) // hello,golang
// 判断字符串是否以指定前缀开头
str4 := "hello,world"
if strings.HasPrefix(str4, "hello") {
fmt.Println("str4 starts with hello")
}
// 判断字符串是否以指定后缀结尾
str5 := "hello,world"
if strings.HasSuffix(str5, "world") {
fmt.Println("str5 ends with world")
}
// 查找字符串中指定子串的位置
str6 := "hello,world"
index := strings.Index(str6, "world")
fmt.Println(index) // 6
}
```
阅读全文
相关推荐
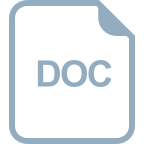
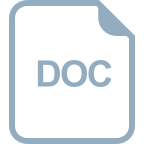
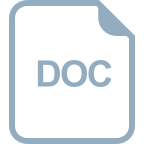

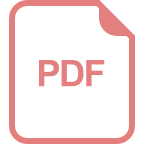
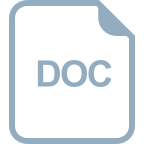
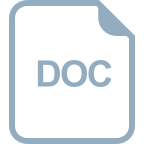

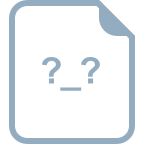
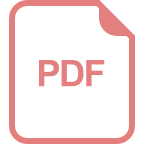
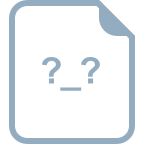
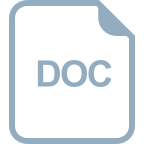
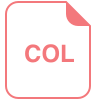
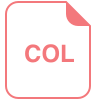
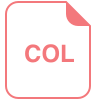
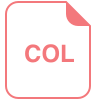
