pycharm仿射密码随机输入一段明文加密或者密文解密
时间: 2023-07-05 18:23:41 浏览: 149
好的,我可以为您提供一些关于仿射密码的代码示例,并且您可以根据需要进行修改。
加密代码:
```
import random
# 定义明文和密文的字符集
plaintext_chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
ciphertext_chars = "XPMGTDHLYONZBWEARKJUFSCIQV"
# 随机生成密钥
a = random.randint(1, 25)
b = random.randint(1, 25)
# 定义仿射密码加密函数
def affine_encrypt(plaintext):
ciphertext = ""
for char in plaintext:
if char in plaintext_chars:
# 明文字符对应的索引
char_index = plaintext_chars.index(char)
# 仿射运算
cipher_index = (a * char_index + b) % 26
# 加密后的密文字符
cipher_char = ciphertext_chars[cipher_index]
ciphertext += cipher_char
else:
ciphertext += char
return ciphertext
# 获取用户输入的明文
plaintext = input("请输入明文:")
# 加密明文并输出
ciphertext = affine_encrypt(plaintext)
print("密文为:", ciphertext)
```
解密代码:
```
# 定义仿射密码解密函数
def affine_decrypt(ciphertext):
plaintext = ""
# 求逆元
a_inverse = 0
for i in range(26):
if (a * i) % 26 == 1:
a_inverse = i
break
for char in ciphertext:
if char in ciphertext_chars:
# 密文字符对应的索引
char_index = ciphertext_chars.index(char)
# 仿射运算的逆运算
plain_index = (a_inverse * (char_index - b)) % 26
# 解密后的明文字符
plain_char = plaintext_chars[plain_index]
plaintext += plain_char
else:
plaintext += char
return plaintext
# 获取用户输入的密文
ciphertext = input("请输入密文:")
# 解密密文并输出
plaintext = affine_decrypt(ciphertext)
print("明文为:", plaintext)
```
这是一个简单的仿射密码实现,您可以根据需要进行修改和扩展。
阅读全文
相关推荐
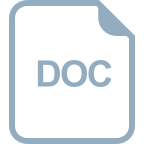
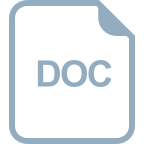
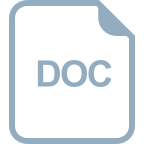



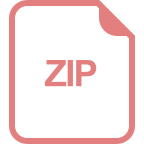
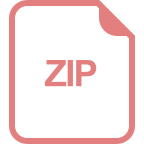
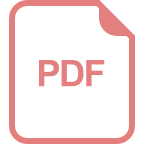
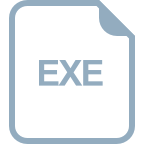
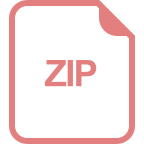
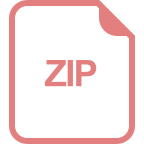
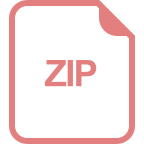
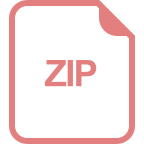
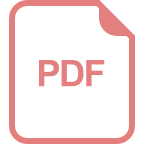
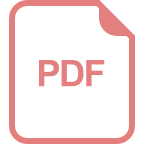

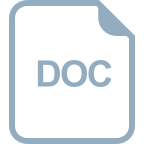